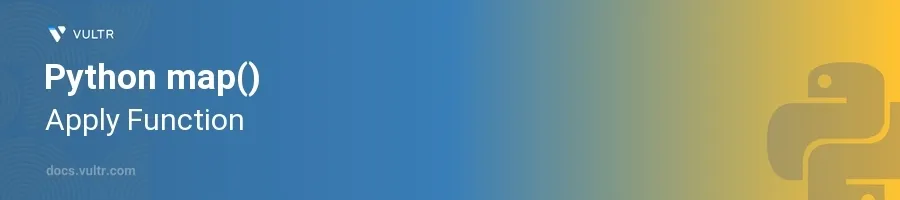
Introduction
The map()
function in Python is a built-in feature that applies a specified function to each item of an iterable, such as a list or tuple, and returns a map object. This functionality can be especially useful for performing transformation operations on data efficiently within a single line of code.
In this article, you will learn how to effectively utilize the map()
function to transform data across various data structures. Explore practical examples that demonstrate transforming lists and combining map()
with other functional programming tools to handle more complex data processing needs.
Applying map() to Lists
Transform Elements in a List
Define a function that specifies the transformation for each element.
Use
map()
to apply this function across an iterable.pythondef square(number): return number ** 2 numbers = [1, 2, 3, 4, 5] squared_numbers = map(square, numbers) print(list(squared_numbers))
This code defines a function
square
that computes the square of a number.map()
applies thissquare
function to each element in thenumbers
list, resulting in a new list of squared numbers.
Convert Data Types in a List
Utilize
map()
to convert all elements of a list to a different data type.pythonstring_numbers = ["1", "2", "3", "4"] int_numbers = map(int, string_numbers) print(list(int_numbers))
Here,
map()
is used to convert each string in the liststring_numbers
to an integer, illustrating the versatility ofmap()
for type conversion in data processing tasks.
Using map() with Other Functions
Apply a Lambda Function
Recognize that lambda functions provide a quick way of defining simple functions.
Use a lambda function directly inside
map()
for concise code.pythonnumbers = [100, 200, 300, 400] halved_numbers = map(lambda x: x / 2, numbers) print(list(halved_numbers))
In this example, a lambda function is used to halve each number in the list. The lambda function provides a compact way to define the operation directly within the
map()
call.
Combine map() with str Methods
Pipe the output of
map()
into string methods to manipulate strings in a list efficiently.pythonnames = ["alice", "bob", "charlie"] capitalized_names = map(str.capitalize, names) print(list(capitalized_names))
Each name in the
names
list is passed to thestr.capitalize
method throughmap()
, capitalizing each name. This example shows howmap()
can be integrated seamlessly with other built-in functions for string manipulation.
Conclusion
The map()
function is a powerful utility in Python that allows for clean, readable, and efficient data transformation operations across iterable data structures. By understanding how to apply map()
to various types of functions and data, you can streamline your code and enhance processing speed. Experiment with integrating map()
with different functions and iterables to maximize your productivity and code efficiency in Python projects.
No comments yet.