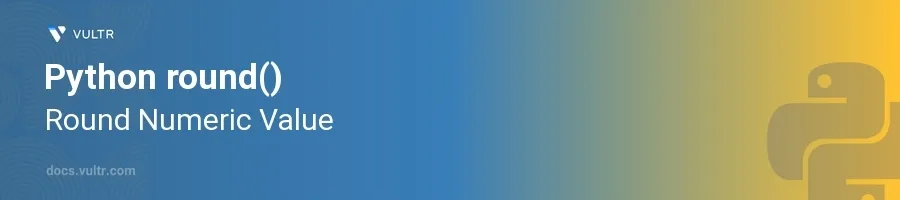
Introduction
The round()
function in Python is a built-in function used to round a number to a specified number of decimal places or to the nearest integer if no number of decimal places is specified. This function is crucial in financial calculations, data analysis, or whenever precise control over numerical precision is needed.
In this article, you will learn how to effectively use the round()
function in various scenarios. Discover how to round numbers to different degrees of precision and understand the behavior of this function under different circumstances.
Rounding to Nearest Integer
Basic Rounding Without Decimal
Start with a floating point number.
Use
round()
to round it to the nearest integer.pythonnumber = 14.7 rounded_number = round(number) print(rounded_number)
This code rounds the number
14.7
to15
. Theround()
function rounds to the nearest even number if the number is exactly halfway between two integers (e.g.,round(2.5)
results in2
).
Rounding Negative Numbers
Take a negative floating point value.
Apply the
round()
function to see the rounding effect on negative numbers.pythonnegative_number = -14.7 rounded_negative = round(negative_number) print(rounded_negative)
Here,
round()
rounds-14.7
to-15
, demonstrating thatround()
functions consistently with negative numbers as well.
Rounding with Specified Decimal Places
Rounding to One Decimal Place
Define a float that has more than one decimal.
Use
round()
to round it to one decimal place.pythondecimal_number = 8.346 rounded_decimal = round(decimal_number, 1) print(rounded_decimal)
This code snippet rounds
8.346
to8.3
. The second argument,1
, specifies the number of decimal places.
Rounding with Multiple Decimal Places
Start with a float with multiple decimal places.
Round it using
round()
to more than one decimal place.pythonprecise_number = 9.87654 rounded_precise = round(precise_number, 3) print(rounded_precise)
This example rounds
9.87654
to9.877
. Specifying3
as the second argument rounds the number to three decimal places.
Special Cases and Considerations
Rounding Halfway Numbers
Understand that Python uses the rounding half to even strategy.
Consider numbers that are exactly halfway between two possible rounded values.
pythonhalfway_number = 2.5 round_halfway = round(halfway_number) print(round_halfway)
The
round(2.5)
results in2
, not3
, due to the half to even rounding rule used by Python'sround()
.
Influence of Zero and Negative Rounding Precision
Realize you can use
0
or negative integers as rounding precision.Observe rounding to tens, hundreds, etc., by specifying negative precision.
pythonlarge_number = 1234.5678 round_negative = round(large_number, -2) print(round_negative)
Here,
1234.5678
is rounded to1200
when using-2
as the decimal places. The negative rounding precision rounds the number to the closest hundred.
Conclusion
The round()
function in Python offers a versatile way to manage numeric precision in your programming tasks. Whether rounding to the nearest integer, specific decimal places, or handling special cases like halfway numbers, round()
provides both simplicity and precision. By mastering the use of this function, you enhance data handling capabilities in your applications, ensuring accuracy where it matters most. Use the examples and tips shared to integrate rounding effectively in your Python solutions.
No comments yet.