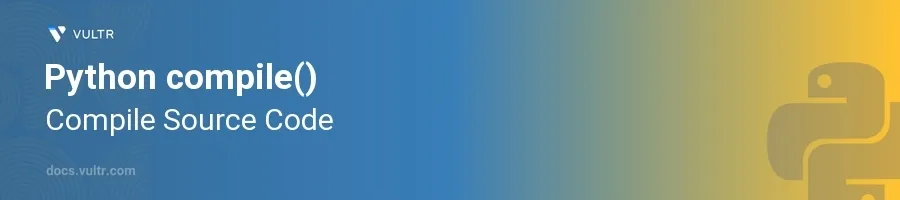
Introduction
The compile()
function in Python is a built-in function that transforms source code into a code or AST object, which can then be executed or evaluated. This function is crucial for applications that need to execute or evaluate Python code dynamically. It provides flexibility in handling code as it allows the creation of executable objects on the fly from strings or AST representations of Python source code.
In this article, you will learn how to use the compile()
function effectively in different contexts. Explore how to compile different types of source information such as strings and AST objects, manage various compilation modes, and execute compiled objects.
Understanding the compile() Function
Basic Usage of compile()
Define a string of Python code.
Use
compile()
to convert the string into a code object.Execute the compiled code using
exec()
oreval()
.pythoncode_str = 'x = 5\nprint("x squared is", x**2)' compiled_code = compile(code_str, 'file_name.py', 'exec') exec(compiled_code)
This snippet demonstrates how to compile a simple piece of code that computes the square of a number and prints it. Here, 'file_name.py' is a filename string which is used for error messages, and 'exec' denotes that the compilation mode is intended for executing statements.
Working with Different Compilation Modes
Understand that
compile()
supports three modes:'exec'
,'eval'
, and'single'
.Use
'exec'
to compile statements,'eval'
for expressions, and'single'
for a single interactive statement.Example for each mode:
python# Exec mode for multiple statements exec_code = compile('name = "Alice"\nprint(name)', 'example.py', 'exec') exec(exec_code) # Eval mode for expressions eval_code = compile('3 + 4', 'expr.py', 'eval') result = eval(eval_code) print("Result of eval:", result) # Single mode for single interactive statement single_code = compile('name = "Bob"\nprint(name)', 'single_stmt.py', 'single') exec(single_code)
This example shows how to compile code using different modes. In the 'eval' mode, only expressions can be compiled and evaluated.
Handling Errors During Compilation
Be aware that syntax errors during compilation will raise a
SyntaxError
.Handle these errors using try-except blocks to make your code more robust.
pythontry: err_code = compile('if True print("Hello")', 'err.py', 'exec') exec(err_code) except SyntaxError as e: print("Caught a SyntaxError:", e)
Here, a SyntaxError occurs due to missing colon (
:
) afterTrue
. The exception is caught and handled gracefully, displaying the error message.
Conclusion
The compile()
function in Python offers a versatile way to dynamically convert Python code into executable objects. Whether it's compiling code from strings, managing different compilation modes, or handling errors effectively, compile()
provides the necessary tools to manage and execute Python code dynamically. By mastering the use of the compile()
function, you enhance your ability to manage code execution in Python scripts dynamically and securely. Use these techniques to handle dynamic code execution needs efficiently and safely in your projects.
No comments yet.