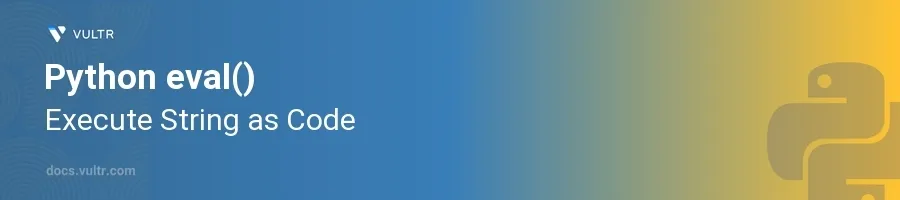
Introduction
The eval()
function in Python evaluates a given string as Python code. This capability is especially powerful as it allows for the execution of Python expressions dynamically. It's particularly useful in scenarios where the code needs to be generated on the fly or when expressions are received as user inputs.
In this article, you will learn how to safely and effectively leverage the eval()
function in various scenarios. You explore practical applications, understand potential risks, and discover how to mitigate those risks while using this function.
Understanding eval()
Basic Usage of eval()
Assign a string that represents a Python expression.
Pass this string to the
eval()
function.pythonexpression = '3 + 4' result = eval(expression) print(result)
This code evaluates the string
expression
which represents the mathematical operation3 + 4
.eval()
calculates this and returns7
.
Evaluating Complex Expressions
Define a string that includes a more complex operation.
Use
eval()
to execute this operation.pythoncomplex_expr = '[num * 2 for num in range(5)]' result = eval(complex_expr) print(result)
Here, the
eval()
function takes a string describing a list comprehension that doubles each number in a range from0
to4
. The output will be[0, 2, 4, 6, 8]
.
Safe Usage of eval()
Risks of Using eval()
Understand that eval()
can execute arbitrary code, which can pose a serious security risk if not handled correctly, especially if the input is taken from untrusted sources.
Mitigating Security Risks
Never use
eval()
with raw inputs from users.Restrict the scope of what
eval()
can access to prevent unintended code execution.pythonfrom math import sqrt user_input = 'sqrt(9)' # Simulate user input allowed_functions = {'sqrt': sqrt} result = eval(user_input, {"__builtins__": None}, allowed_functions) print(result)
This snippet demonstrates how to restrict
eval()
to only access specific functions by defining a limited dictionary of allowed functions and passing it as the environment. This prevents potentially harmful operations.
Practical Examples of eval()
Dynamic Function Dispatch
Create a dictionary mapping commands to their corresponding functions.
Use
eval()
to execute a function based on user input safely.pythondef greet(name): return "Hello, " + name actions = {'greet': 'greet("Alice")'} user_command = 'greet' # Simulated user command result = eval(actions[user_command], {"__builtins__": None}, {'greet': greet}) print(result)
This example maps a user command to a function call, ensuring that only predefined commands are executable, thus maintaining control over the execution environment.
Conclusion
The eval()
function in Python is a highly flexible tool that allows for the execution of Python code contained within strings. While it opens up many dynamic programming possibilities, it also introduces significant security risks. Always handle eval()
with care, particularly when dealing with external inputs. By following the practices outlined, you ensure that your use of eval()
is both powerful and secure, allowing for creative yet safe Python programming solutions.
No comments yet.