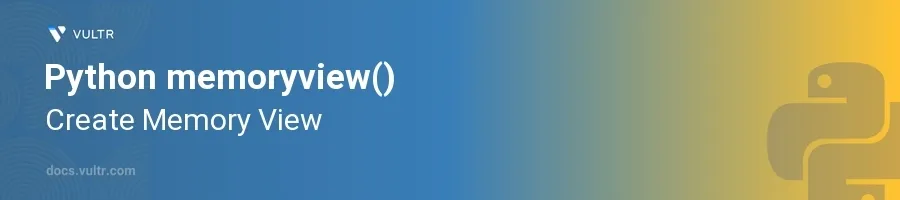
Introduction
The memoryview()
function in Python provides a way to access the memory of an object without copying its contents. This is particularly useful for handling slices of arrays and manipulating large datasets without the overhead of copying the data. The memoryview()
function returns a memory view object from a specified object, which supports the buffer protocol.
In this article, you will learn how to create and manipulate memory views in Python using the memoryview()
function. Explore how this function can be applied to bytes, bytearray, and other objects to efficiently handle data operations.
Creating a Memory View in Python
Creating a Memory View of Bytes
Construct a bytes object.
Generate a memory view from the bytes object.
pythonbytes_obj = b'Hello, World!' mem_view = memoryview(bytes_obj) print(mem_view)
This code creates a memory view of the
bytes_obj
. Thememoryview()
function takes the bytes object as an argument and returns a view of its memory.
Creating a Memory View of a Bytearray
Create a mutable bytearray.
Obtain a memory view of the bytearray.
pythonbytearray_obj = bytearray(b'Example data') mem_view = memoryview(bytearray_obj) print(mem_view)
Here, a memory view is created from
bytearray_obj
. This allows direct, buffer-level access to the bytearray contents.
Manipulating Data Using Memory View
Slicing a Memory View
Understand that slices of memory views return new memory views of the slice, not copies of the data.
Slice the memory view to access a part of the data.
pythonmem_slice = mem_view[7:12] print(mem_slice.tobytes())
Slicing the memory view object
mem_view
retrieves a slice from index 7 to 11. The.tobytes()
method converts the memory view slice back to bytes for display.
Modifying Data Through Memory View
Keep in mind that modifications to the memory view affect the original data when the base object is mutable.
Modify an element in the memory view of a bytearray.
pythonmem_view[0] = 65 # 'A' in ASCII print(bytearray_obj)
Modifying the memory view directly updates the original
bytearray_obj
, demonstrating how memory views facilitate efficient data manipulation.
Conclusion
The memoryview()
function in Python offers a practical tool for accessing and manipulating the memory of various objects without copying their contents. It is especially valuable in scenarios where performance is critical and large data sets are used. By creating memory views, you gain buffer-level access to the data, allowing for efficient and effective data manipulation processes. Utilize the techniques discussed here to work more adeptly with bytes and bytearrays, and leverage the power of direct memory access in your Python applications.
No comments yet.