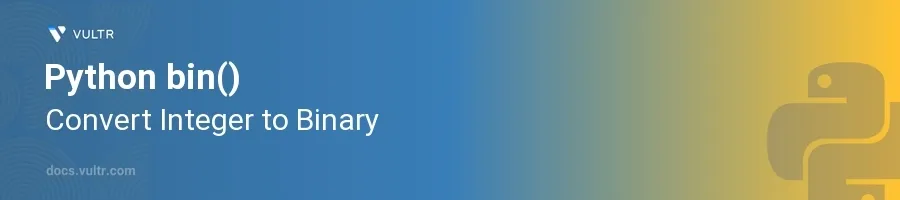
Introduction
The bin()
function in Python is a built-in utility used to convert an integer into its corresponding binary representation, prefixed with '0b', which indicates a binary value. This function becomes particularly useful in fields dealing with low-level programming, bit manipulation, or when simply needing to understand the binary form of numbers for debugging purposes.
In this article, you will learn how to use the bin()
function to convert integers to binary strings. The discussion includes converting both positive and negative integers, and how to manipulate or format the resulting binary strings for various applications.
Basic Usage of bin()
Convert a Positive Integer to Binary
Choose a positive integer to convert.
Apply the
bin()
function to the integer.pythonpositive_number = 14 binary_representation = bin(positive_number) print(binary_representation)
This snippet will convert the integer
14
to its binary form, which outputs0b1110
.
Convert a Negative Integer to Binary
Select a negative integer for conversion.
Use the
bin()
function on this integer.pythonnegative_number = -14 binary_representation = bin(negative_number) print(binary_representation)
For negative numbers, Python uses two's complement notation. In this case,
-14
is output as-0b1110
.
Advanced Manipulations with bin()
Removing the '0b' Prefix
Convert an integer to binary.
Use string slicing to remove the '0b' prefix.
pythonnumber = 14 binary_representation = bin(number)[2:] print(binary_representation)
Removing the
0b
makes the output simply1110
, which might be necessary for certain formatting requirements or calculations.
Formatting Binary Output for Fixed Width
Convert the integer to binary, removing the prefix.
Use string formatting to pad the binary string to a fixed width, using zeroes.
pythonnumber = 5 formatted_binary = format(number, '08b') print(formatted_binary)
Here, the binary representation of
5
is formatted to a width of 8, resulting in00000101
. This is useful in scenarios where binary values need to be of consistent length, like byte-aligned data manipulation.
Conclusion
The bin()
function in Python offers a straightforward method to convert integers into their binary representations. Whether handling positive or negative integers, the function provides clear, two's-complement formatted binary strings accurately and efficiently. Use bin()
in a wide array of applications, from simple data representation transformations to more complex binary data processing tasks. By understanding and applying the techniques discussed, you enhance the flexibility and power of your programming toolkit for any projects that require binary data manipulation.
No comments yet.