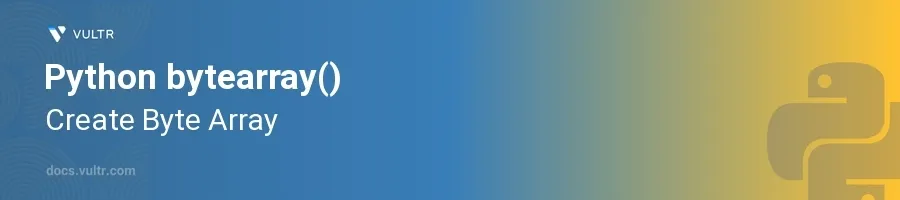
Introduction
The bytearray()
function in Python is integral for working with mutable sequences of integers, which are commonly used to store binary data. Unlike bytes
, bytearray objects are changeable, allowing modifications to individual elements or efficient appending of new data. These features make bytearray useful in applications where direct manipulation of binary data is required, such as file I/O, network communications, and low-level system interfacing.
In this article, you will learn how to effectively utilize the bytearray()
function in Python to create and manipulate byte arrays. Explore different ways to initialize byte arrays, modify existing data, and use them in real-world applications, enhancing your proficiency with binary data operations.
Creating Byte Arrays Using bytearray()
Direct Initialization with Strings
Initialize a bytearray from a string by specifying an encoding. The encoding is necessary to convert the string into bytes.
pythonexample_string = "Hello, world!" byte_array = bytearray(example_string, encoding='utf-8') print(byte_array)
This code snippet creates a bytearray from a string, using UTF-8 encoding. The
print
statement will show the bytearray representation of "Hello, world!".
Creating from an Iterable of Integers
Generate a bytearray from an iterable of integers, where each integer represents a valid byte (0-255).
pythoninteger_list = [65, 66, 67] byte_array = bytearray(integer_list) print(byte_array)
Here,
byte_array
is initialized with elements corresponding to ASCII values for 'A', 'B', and 'C'. When printed, it displays the bytes representing these characters.
Initializing with a Given Size
Form a bytearray of a specified size initialized with null bytes. This method is useful when you need a buffer of a fixed size.
pythonsize = 10 byte_array = bytearray(size) print(byte_array)
The resulting
byte_array
consists of ten bytes, all set to0
. It can be used as a buffer in operations like reading from a file or socket in specified chunks.
Manipulating Byte Arrays
After initializing a bytearray, modifying its content is straightforward due to its mutable nature.
Modifying Individual Bytes
Access the bytearray by its indices to change specific bytes.
pythonbyte_array = bytearray("Hello", 'utf-8') byte_array[0] = 72 # 'H' in ASCII byte_array[1] = 101 # 'e' in ASCII print(byte_array)
This example initially creates a bytearray from the string "Hello" and then modifies the first two elements. The print output will still show "Hello" as it simply reaffirms the initial values.
Appending Data
Use the
.append()
or.extend()
methods to add more data to the bytearray.pythonbyte_array = bytearray([72, 101]) byte_array.append(108) # Append ASCII for 'l' byte_array.extend([108, 111]) # Append ASCII for 'l' and 'o' print(byte_array.decode('utf-8'))
This code appends characters 'l' and 'o' to initially stored 'He', finally resulting in the string "Hello" upon decoding.
Conclusion
The bytearray()
function in Python provides a flexible tool for manipulating binary data interactively. By understanding how to create, modify, and extend bytearrays, you can handle binary data more effectively in your Python applications. Utilize the bytearray functionalities to read, modify, and write binary data structures efficiently, ensuring your operations on mutable byte arrays are robust and performant. Incorporate these methods in networking tasks, file handling, or any scenario that requires direct binary data manipulation.
No comments yet.