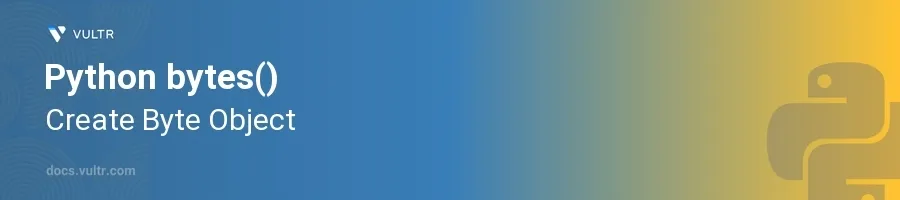
Introduction
The bytes()
function in Python is essential for dealing with byte data, which is immutable sequences of single bytes. Often used in network communications, file handling, and interfacing with binary data, the bytes()
function is a fundamental tool in Python programming for handling such information efficiently.
In this article, you will learn how to create and manipulate byte objects using the bytes()
function. Explore how to convert different data types into bytes, including strings, integers, and iterable objects, and understand the implications of using this function in data processing and manipulation tasks.
Creating Byte Objects Using bytes()
Creating Empty Byte Objects
Use
bytes()
without any arguments to create an empty byte object.pythonempty_bytes = bytes() print(empty_bytes)
This code snippet initializes an empty byte object. It's particularly useful when you need a starting point for building byte data progressively.
From Integer Values
Pass an integer to
bytes()
to create a byte object of that length initialized with null bytes.pythonsize_bytes = bytes(10) print(size_bytes)
Here,
bytes(10)
creates a byte object consisting of ten bytes, all initialized to zero (null
bytes). This method is commonly used when a specific size of byte data is required.
From Iterable of Integers
Provide an iterable of integers (0-255) to
bytes()
.pythonlist_bytes = bytes([65, 66, 67]) print(list_bytes)
Each integer in the iterable is converted to the corresponding byte value. In this example, the ASCII values for 'A', 'B', and 'C' are converted into byte representation.
Converting Strings to Bytes
Simple Conversion
Convert a string to bytes using
bytes()
with encoding.pythonstring = "Hello" encoded_string = bytes(string, 'utf-8') print(encoded_string)
By specifying the encoding type ('utf-8' in this case), you can convert a string into its bytes format. This conversion is crucial for file writing in binary mode or network data transmission.
Manipulating Byte Objects
Once you create byte objects, remember that they are immutable. To modify byte data, convert it into a bytearray
, which allows for mutable operations.
Convert bytes to bytearray and change its content.
pythonmutable_bytes = bytearray(b"Hello") mutable_bytes[0] = 104 # Change 'H' to 'h' print(mutable_bytes)
This snippet shows how to make a byte object mutable by using
bytearray
, allowing modifications like changing specific bytes.
Conclusion
The bytes()
function in Python is a versatile tool for handling binary data efficiently. By understanding how to create byte objects from various data types and manipulate them appropriately, you enhance your ability to work with file systems, networks, and other binary interfaces. Whether starting with basic byte operations or dealing with complex data transformations, mastering the bytes()
function paves the way for effective and efficient Python programming in tasks involving binary data handling.
No comments yet.