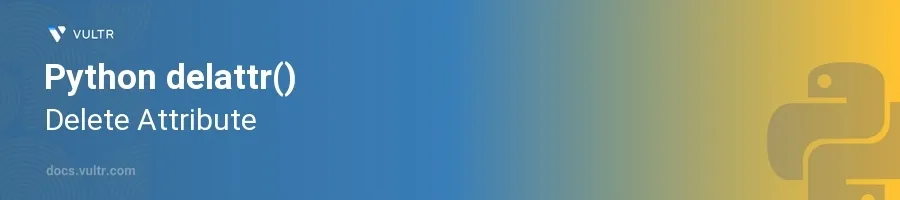
Introduction
The delattr()
function in Python serves a critical role in dynamic attribute management by enabling the deletion of an attribute from an object. This capability is particularly useful in scenarios involving mutable or configurable objects where attributes might need to be removed during runtime based on specific conditions or configurations.
In this article, you will learn how to effectively use the delattr()
function to manipulate object attributes dynamically. Explore various practical applications, including handling custom object attributes and ensuring safe attribute deletion to prevent runtime errors.
Basics of delattr()
Deleting an Attribute from an Object
Define a custom class with several attributes.
Use
delattr()
to remove a specific attribute.pythonclass Vehicle: def __init__(self, make, model, year): self.make = make self.model = model self.year = year car = Vehicle('Toyota', 'Corolla', 2020) delattr(car, 'model')
This code defines a
Vehicle
class with attributes likemake
,model
, andyear
. Thedelattr()
function then deletes themodel
attribute from thecar
instance.
Handling Exceptions When Deleting Attributes
Understand that trying to delete a non-existing attribute raises an
AttributeError
.Implement error handling using a try-except block to manage this scenario.
pythoncar = Vehicle('Honda', 'Civic', 2019) try: delattr(car, 'color') except AttributeError: print("The attribute does not exist.")
In this example, since 'color' is not an attribute of
car
, attempting to delete it raises anAttributeError
, which is then caught by the except block.
Advanced Usage of delattr()
Dynamically Removing Attributes in a Data-Cleaning Process
Work with instances where attributes might be conditionally removed based on data analysis or preprocessing needs.
Perform attribute removal based on condition checks.
pythonimport random class Data: def __init__(self): self.age = random.randint(10, 100) self.name = "Unknown" self.valid = random.choice([True, False]) data_record = Data() if not data_record.valid: delattr(data_record, 'age')
Here,
delattr()
is used to remove theage
attribute ifdata_record.valid
isFalse
. This might be part of a preprocessing step where invalid data records are stripped of certain attributes.
Implementing Conditional Deletion in a Loop
Iterate over a list of objects and delete attributes based on a specific condition or value.
Use
delattr()
within a loop to streamline attribute management in multiple objects.pythonclass Product: def __init__(self, identifier, price, discontinued): self.id = identifier self.price = price self.discontinued = discontinued inventory = [Product('001', 15.99, False), Product('002', 23.50, True)] for item in inventory: if item.discontinued: delattr(item, 'price')
The provided code iterates through a list of
Product
instances. If the product is discontinued, the price attribute is deleted to reflect its updated status in inventory management.
Conclusion
Harness the functionality of delattr()
in Python to dynamically manage attributes of objects. This functionality fosters flexibility in how data objects are modified and managed at runtime, essential for applications that require dynamic configuration or adjustment of their properties. Through careful implementation of delattr()
, coupled with robust error handling, enhance the adaptability and resilience of Python applications across diverse operational scenarios.
No comments yet.