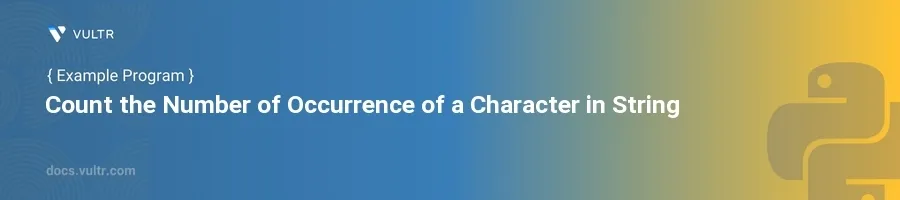
Introduction
Determining the frequency of characters in a string is a common task in software development, especially in fields like data analysis, cryptography, and text processing. This functionality can be utilized to understand patterns, encode messages, or simply manipulate text data more effectively.
In this article, you will learn how to count the number of occurrences of a character in a string using Python. Explore practical code examples that illustrate simple yet efficient methods to achieve this goal, whether you're dealing with large datasets or just automating daily tasks.
Counting Character Occurrences
Using the count() Method
Utilize Python’s built-in string method
count()
to find how many times a specific character appears in a string.Define a string and the character you want to count.
Apply the
count()
method to get the number of occurrences.pythonsample_text = "hello world" character_to_count = 'o' count = sample_text.count(character_to_count) print(count)
This code counts how many times the character 'o' appears in
sample_text
. It outputs the count, which is 2 in this example.
Employing a for Loop and a Dictionary
Use a for loop to iterate through each character in the string.
Increment the character's count in a dictionary each time it occurs.
Print the number of times the specified character appears.
pythonsample_text = "hello world" character_to_count = 'l' frequency = {} for char in sample_text: if char in frequency: frequency[char] += 1 else: frequency[char] = 1 print(frequency.get(character_to_count, 0))
This snippet creates a dictionary
frequency
that maps each character to its number of occurrences. It prints the count of 'l', which appears 3 times insample_text
.
Using a List Comprehension and sum()
Combine Python’s
sum()
function and a list comprehension to count occurrences compactly.Check each character in the string and compare it to the target character.
Sum up all matches to get the total count.
pythonsample_text = "hello world" character_to_count = 'r' count = sum(1 for char in sample_text if char == character_to_count) print(count)
By using
sum()
with a generator expression, this approach sums 1 for every instance wherechar
equals 'r'. This method confirms 'r' appears once in thesample_text
.
Conclusion
Counting the number of occurrences of a character in a Python string can be approached in various ways, depending on your specific needs and preference for code clarity. From using the straightforward count()
method to implementing a loop with a dictionary or utilizing list comprehensions, each technique offers a suitable solution for different scenarios. With these methods in your toolkit, you are well-equipped to handle tasks involving text manipulation and analysis, ensuring your programs are both effective and efficient.
No comments yet.