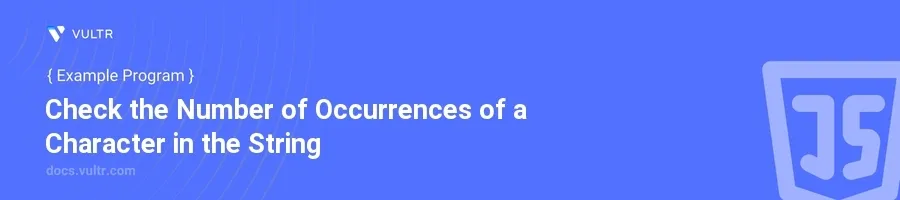
Introduction
When working with text data in JavaScript, you might often need to determine how frequently a character appears in a string. This task is fundamental in many applications such as data validation, search functionality, and text analysis. Counting the occurrences of a character helps in understanding the composition of the text, checking for typos, or even in cryptographic analysis.
In this article, you will learn how to count the number of times a specific character appears in a string in JavaScript. You will see practical examples that demonstrate the use of simple loops, the split()
method, and newer ES6 features like the reduce()
method for achieving this task.
Counting Character Occurrences
Using a For Loop
Initialize a counter to keep track of occurrences.
Loop through each character in the string.
Increment the counter each time the character matches the target character.
javascriptfunction countCharacter(str, char) { let count = 0; for (let i = 0; i < str.length; i++) { if (str[i] === char) { count++; } } return count; } console.log(countCharacter("hello world", "l")); // Output: 3
This snippet iterates over the string
"hello world"
and counts how many times the character"l"
appears.
Using the Split Method
Split the string into an array of substrings using the target character as a separator.
The occurrence of the character is one less than the length of the resulting array.
javascriptfunction countCharacterSplit(str, char) { return str.split(char).length - 1; } console.log(countCharacterSplit("hello world", "o")); // Output: 2
Here, splitting
"hello world"
by"o"
results in an array with two elements:["hell", " world"]
. The character"o"
appears 2 times.
Using the Reduce Method
Convert the string into an array using the
split()
method.Use the
reduce()
method to accumulate a count of the target character.javascriptfunction countCharacterReduce(str, char) { return str.split('').reduce((count, currentChar) => { return currentChar === char ? count + 1 : count; }, 0); } console.log(countCharacterReduce("hello world", "l")); // Output: 3
In this code,
reduce()
traverses each character in the array, incrementally counting occurrences of"l"
.
Conclusion
Counting the number of occurrences of a character in a string is a common task that can be accomplished in JavaScript using several different methods. Whether you choose a traditional for loop, utilize the string split()
method for a more functional approach, or leverage the powerful reduce()
method, each technique has its own use-case depending on the context of the problem and personal or project-specific coding standards. With the examples provided, tackle any scenario requiring you to count characters with confidence and efficiency.
No comments yet.