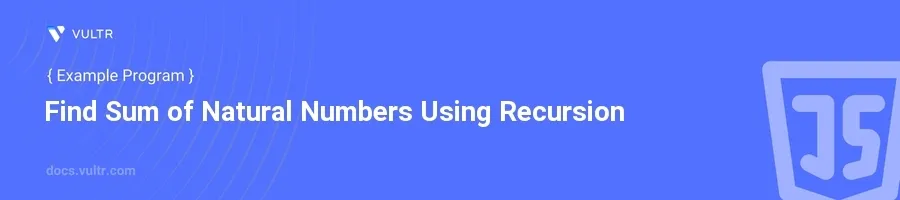
Introduction
Recursion in programming refers to the technique where a function calls itself directly or indirectly to solve a problem. This method is often used for solving problems that can be broken down into similar sub-problems. JavaScript, being a versatile language, supports recursion and can use this concept to efficiently perform tasks like calculating the sum of natural numbers.
In this article, you will learn how to write a recursive function in JavaScript to calculate the sum of the first 'n' natural numbers. Discover the structure of recursive functions and deepen your understanding through practical code examples.
Understanding Recursion with a Simple Example
Recursive Function for Sum of Natural Numbers
Define a function that takes one parameter,
n
, which represents the number of first natural numbers to sum.Implement the recursive logic to perform the addition.
javascriptfunction sumNaturalNumbers(n) { if (n <= 0) { return 0; } else { return n + sumNaturalNumbers(n - 1); } }
In this function:
- The base case is when
n
is less than or equal to zero. Here, the function returns0
. - The recursive case is when
n
is greater than zero. The function calls itself withn-1
and addsn
to the result of the recursive call.
- The base case is when
Illustrating the Recursive Calls
Understand how the function call works using a smaller value for ease.
javascriptconsole.log("Sum of first 5 natural numbers is:", sumNaturalNumbers(5));
The function calls will unfold as follows:
sumNaturalNumbers(5)
will return5 + sumNaturalNumbers(4)
sumNaturalNumbers(4)
will return4 + sumNaturalNumbers(3)
- Continues until
sumNaturalNumbers(0)
returns0
Analyzing the Behavior of the Function
Characteristics of Recursive Solutions
- Understand that every recursive function must have a base case to avoid infinite loops.
- Recognize that the function reduces the problem size with each recursive call until it reaches the base case.
- Observe that recursive functions can sometimes lead to performance issues like stack overflow if the input size is large.
Performance Implications
- Note that recursive solutions are not always the most efficient in terms of time and space complexity.
- Acknowledge that iterative solutions might offer better performance for problems like summing natural numbers, though recursion shines in readability and simplicity for many cases.
Conclusion
Recursion is a powerful concept in JavaScript for solving problems that can be decomposed into smaller instances of the same problem. The sum of natural numbers is a classic example to understand the mechanics behind recursive functions. By breaking down the problem and solving it recursively, one can gain a deeper insight into both the problem and the recursion technique. Although recursion offers an elegant solution, always consider the implications on performance and choose the right approach based on the problem context.
No comments yet.