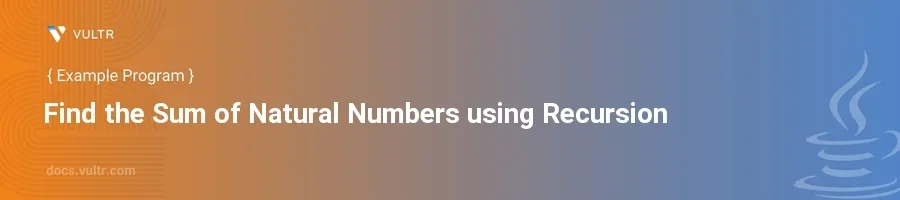
Introduction
Recursion in Java is a process in which a method calls itself continuously. A method in Java that calls itself is called a recursive method. It's a powerful concept that can solve complex problems with less code compared to iterative approaches. In particular, calculating the sum of natural numbers becomes conceptually simple using recursion.
In this article, you will learn how to utilize recursion for calculating the sum of natural numbers. Explore the concept through detailed examples and understand how to incorporate recursion effectively within your Java programs for similar tasks.
Understanding Recursion
Recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. Before jumping into constructing a recursive Java program for the sum of natural numbers, let’s briefly look at what constitutes a well-defined recursive function:
- Base Case: The condition under which the recursion stops.
- Recursive Case: The part of the function that calls itself.
This approach not only simplifies the code but also aids in breaking down complex tasks into simpler sub-tasks.
Writing the Recursive Function
Create the Recursive Method
Define a Java method named
sum
that takes an integern
as its parameter. This parameter represents the number at which the summation starts counting downwards to 1.Implement the base case. If
n
is 1, return 1 because the sum of the first natural number is itself.Implement the recursive case where the method calls itself with
n-1
and addsn
to the result of this call.javapublic class NaturalSumCalculator { public static int sum(int n) { if (n == 1) { return 1; // Base case } else { return n + sum(n - 1); // Recursive case } } }
In this implementation,
sum
is a method that takes an integern
and returns the sum of all natural numbers up ton
. Ifn
is 1, it simply returns 1, fulfilling the base case. For all other values, it addsn
to the sum of all numbers less thann
, which is obtained by a recursive call to itself withn-1
.
Implementing the Main Method
Create the
main
method within the same class to run and test thesum
method.Call the
sum
method using different values and print the results to see the method in action.javapublic static void main(String[] args) { int result = sum(10); // Calculate sum of first 10 natural numbers System.out.println("Sum of first 10 natural numbers is: " + result); }
This block of code calls the
sum
method with the argument 10, which calculates the sum of numbers from 1 to 10 using recursion, and then prints it out.
Conclusion
Using recursion to find the sum of natural numbers simplifies the logic and implementation. While recursion can lead to elegant solutions as seen in this Java example, be mindful of its memory use and the potential to cause a stack overflow if the recursion depth is too high (e.g., trying to sum a very large sequence of numbers). Nevertheless, by mastering recursive functions like this, you enhance your ability to think critically and solve problems efficiently in Java or any other programming language. By employing the recursive techniques outlined in this article, you ensure your approach to problem-solving remains robust and effective.
No comments yet.