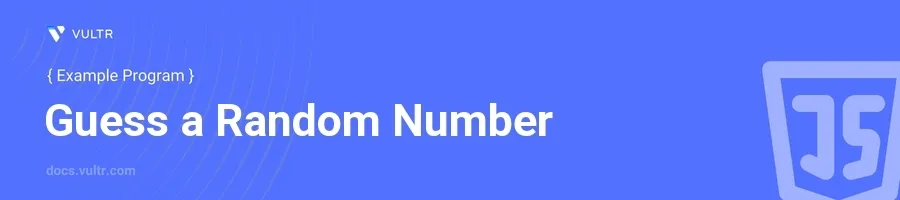
Introduction
Guessing a random number in JavaScript is a common beginner project that demonstrates basic programming concepts such as variables, loops, and user input handling. These kinds of programs not only help in understanding the syntax but also in grasping how logic is built in programming.
In this article, you will learn how to create a simple JavaScript program that generates a random number which the user tries to guess. You'll be guided through different methods and variations to enhance the program’s functionality and provide a more engaging user experience.
Basic Number Guessing Game
Generate a Random Number
First, define the range for the random number. For simplicity, let it be between 1 and 100.
Use the
Math.random()
function to generate the random number.javascriptconst max = 100; const randomNum = Math.floor(Math.random() * max) + 1;
This code generates a random number between 1 and 100.
Math.random()
creates a floating-point number between 0 (inclusive) and 1 (exclusive).Math.floor()
is used to round down to the nearest whole number.
Prompt User to Guess the Number
Prompt the user to guess the number using
prompt()
, which displays a dialog box that asks the user for input.Convert the user input, which is a string, to a number.
javascriptlet guess = parseInt(prompt("Guess the number between 1 and 100:"));
This line of code will show a prompt dialog box where the user can type their guess.
Check the Guess
Use a while loop to allow multiple attempts until the correct guess is made.
Compare the user's guess with the generated number and provide feedback.
javascriptwhile(guess !== randomNum) { if(guess > randomNum) { guess = parseInt(prompt("Too high! Guess again:")); } else { guess = parseInt(prompt("Too low! Guess again:")); } } alert("Congratulations! You guessed the number!");
This loop continues asking the user for guesses until the correct number is guessed. It provides feedback if the guess is too high or too low.
Enhancing the Game
Limiting Number of Guesses
Add a counter to limit the number of guesses the user can make.
javascriptlet guessesLeft = 10; while(guess !== randomNum && guessesLeft > 0) { if(guess > randomNum) { guessesLeft--; guess = parseInt(prompt("Too high! Guess again. You have " + guessesLeft + " guesses left.")); } else { guessesLeft--; guess = parseInt(prompt("Too low! Guess again. You have " + guessesLeft + " guesses left.")); } } if(guess === randomNum) { alert("Congratulations! You guessed the number!"); } else { alert("Out of guesses! The number was " + randomNum); }
This code gives the user a limited number of guesses (10 in this case) to identify the number. It decreases the count with each incorrect guess and notifies how many guesses are left.
Conclusion
Creating a number guessing game in JavaScript is a straightforward project that helps reinforce fundamental concepts like loops, conditions, and user input. By starting with a basic version and then introducing modifications such as guess limits, the game becomes more challenging and engaging, providing not just learning but also a fun experience. Adapt these blocks to craft games with different rules and complexity, enhancing both coding skills and logical thinking.
No comments yet.