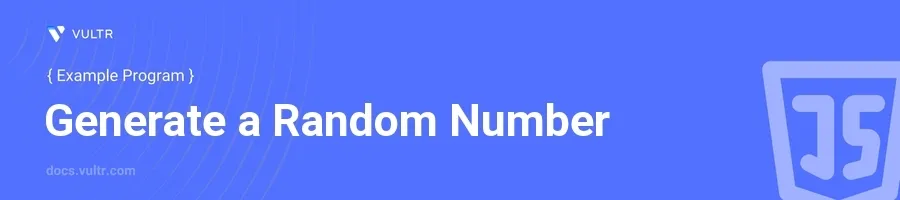
Introduction
JavaScript provides developers with various ways to generate random numbers, which are incredibly useful in scenarios like creating random tests, games, or even in simulations. The ability to incorporate randomness to any part of your code allows for more dynamic applications and models.
In this article, you will learn how to generate random numbers in JavaScript using different methods. Follow through the examples provided to understand the application of randomness in JavaScript programming.
Generating a Random Number Between 0 and 1
Basic Random Number
Use the
Math.random()
function, which generates a random decimal number between 0 (inclusive) and 1 (exclusive).javascriptvar randomNumber = Math.random(); console.log(randomNumber);
The
Math.random()
function returns a floating-point, pseudo-random number which you can use directly for operations that need random decimal values between 0 and 1.
Generating a Whole Number Within a Range
Custom Range Random Number
Define the desired minimum and maximum values.
Adjust the output of
Math.random()
to fit your range.javascriptfunction getRandomNumber(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } var randomNumber = getRandomNumber(10, 50); console.log(randomNumber);
This function,
getRandomNumber
, generates a random whole number between any two specified values, inclusive.Math.floor()
is used to ensure the random number is a whole number.
Ensuring Even Distribution
Random Number with Even Distribution
Understand the importance of even distribution in generating random numbers for unbiased results.
Use a similar approach but with additional checks or a different method to ensure distribution.
javascriptfunction getRandomEvenNumber(min, max) { var result = Math.floor(Math.random() * (max - min + 1)) + min; return result % 2 === 0 ? result : result + 1; } var evenRandomNumber = getRandomEvenNumber(10, 50); console.log(evenRandomNumber);
Here, the function adjusts if the result is odd, ensuring the result is an even number. If it's already even, it remains unchanged.
Conclusion
Generating random numbers in JavaScript is straightforward with the Math.random()
function. By using this function in combination with Math.floor()
, it's possible to create randomly generated integers within a specified range. Custom functions like the ones you've seen here can cater to more specific requirements such as ensuring even distribution or fitting within a custom range. Use these techniques in your applications to add complexity and variability in a controlled manner.
No comments yet.