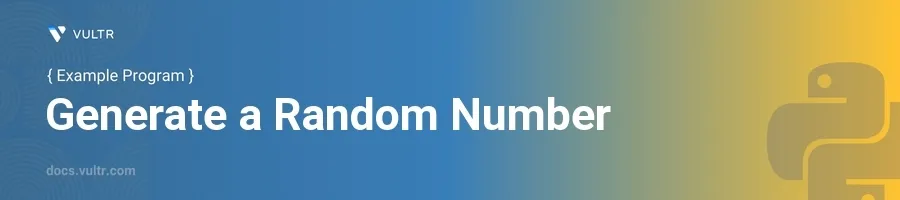
Introduction
Random number generation is an essential aspect of programming that can be used in numerous applications such as simulations, games, testing algorithms, and more. Python provides several ways to generate random numbers through its standard library modules such as random
and numpy
. These tools offer a wide range of functions that can produce random numbers in various distributions and forms.
In this article, you will learn how to generate random numbers in Python using practical examples. Dive into the usage of the random
module for generating random integers and floats, and explore techniques to create random numbers with specific properties such as within a certain range or following a particular distribution.
Generating Random Numbers Using the random
Module
Generate a Basic Random Integer
Import the
random
module.Use the
randint
function to generate a random integer within a specified range.pythonimport random random_integer = random.randint(1, 10) print(random_integer)
This code generates a random integer between 1 and 10, inclusive. The
randint
function takes two parameters, the lower and upper limits of the range, making it ideal for applications requiring an integer within a fixed range.
Generate a Random Float
Use the
random
function to generate a random float.pythonrandom_float = random.random() print(random_float)
Here, the
random
function returns a float number between 0.0 and 1.0. This is useful when you need a random decimal for percentages and probability operations.
Random Element from a List
Use the
choice
function to select a random element from a list.pythoncolors = ['red', 'blue', 'green', 'yellow'] random_color = random.choice(colors) print(random_color)
The array
colors
contains several strings representing colors.choice
randomly selects and returns one of these elements, enabling random selection from any list.
Advanced Random Number Generation
Generate Numbers with randrange
Generate a number from a range by steps using
randrange
.pythonrandom_step = random.randrange(0, 101, 10) print(random_step)
The function
randrange
provides more control over the generated numbers. In the snippet above, it generates a number from 0 to 100 in increments of 10. This can be especially relevant for scenarios requiring random selections from a defined set of intervals.
Using Random for Multiple Choices
Use
sample
to pick multiple unique elements from a list without replacement.pythonlottery_numbers = random.sample(range(1, 50), 5) print(lottery_numbers)
sample
pulls unique entries defined by the second parameter, here choosing 5 unique numbers from 1 to 49. This is ideal for lottery simulations or scenarios where non-repetitive random selection is critical.
Shuffle Elements Randomly
Employ
shuffle
to rearrange items in a list in random order.pythondeck = list(range(1, 53)) random.shuffle(deck) print(deck)
shuffle
modifies the list in-place, making it suited for games or applications needing to mix items or attributes randomly, such as shuffling a deck of cards.
Conclusion
Random number generation in Python is facilitated by the powerful random
module, which offers a range of functions to cater to different randomization needs. From generating simple random integers and floats to more complex distributions and selections, understanding these utilities enhances the capability to implement randomness effectively in programs. Apply these examples as foundations and adapt them to fit the specific requirements of your projects, ensuring reliable and varied random outcomes.
No comments yet.