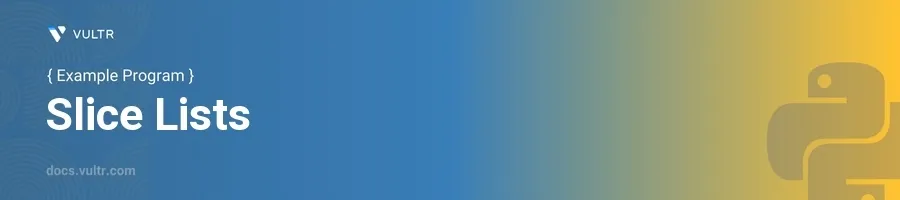
Introduction
Slicing lists in Python is a fundamental technique that enables you to extract portions of a list. This capability is extremely useful in data manipulation and analysis, allowing you to access and modify subsets of list data efficiently. Understanding how to slice lists effectively can enhance your data handling capabilities in Python significantly.
In this article, you will learn how to slice lists in Python through practical examples. Explore the versatility of list slicing with different step values, negative indices, and the use of slicing for list modification and copying.
Basic List Slicing
Understanding the Slicing Syntax
Learn the basic slicing syntax:
[start:stop:step]
, where:start
is the index to begin the slice (inclusive).stop
is the index to end the slice (exclusive).step
specifies the interval between indices.
Start with a simple list and extract a part of it.
pythonexample_list = ['a', 'b', 'c', 'd', 'e'] sliced_part = example_list[1:4] print(sliced_part)
This code will output
['b', 'c', 'd']
. It starts slicing from index 1 up to but not including index 4.
Using Negative Indices
Utilize negative indices to slice from the end of the list.
Try slicing the last three elements of a list.
pythonexample_list = ['apple', 'banana', 'cherry', 'date', 'elderberry'] sliced_negative = example_list[-3:] print(sliced_negative)
The output will be
['cherry', 'date', 'elderberry']
. This slice starts from the third last element to the end of the list.
Skipping Elements with Step
Use the
step
parameter to skip elements in a list slice.Create a slice that includes every second element.
pythonexample_list = [10, 20, 30, 40, 50, 60, 70] skipped_elements = example_list[::2] print(skipped_elements)
Expect
skipped_elements
to show[10, 30, 50, 70]
, skipping one element after each included element.
Advanced Slicing Techniques
Reversing a List with Slicing
Use slicing to reverse a list efficiently.
Apply the technique to print a list in reverse order.
pythonexample_list = [1, 2, 3, 4, 5] reversed_list = example_list[::-1] print(reversed_list)
The result will be
[5, 4, 3, 2, 1]
. This slice goes through the list in reverse.
Combining Slices and List Methods
Combine list slicing with list methods like
append()
orextend()
to modify lists.Extract a portion of a list and append it to another list.
pythonlist_one = ['a', 'b', 'c'] list_two = ['x', 'y', 'z'] portion = list_one[1:] list_two.extend(portion) print(list_two)
This results in
list_two
being['x', 'y', 'z', 'b', 'c']
, where the portion['b', 'c']
fromlist_one
is appended.
Conclusion
Slicing lists in Python is a powerful technique not only for accessing elements but also for manipulating data structures efficiently. With the flexibility of slicing syntax – inclusive of start, stop, and step arguments – and its combination with other list operations, it suits various programming needs. By mastering these slicing methods, you elevate your Python programming skills, especially in applications involving data manipulation and analysis. Keep experimenting with different slices to discover the most efficient ways to handle and transform your data lists.
No comments yet.