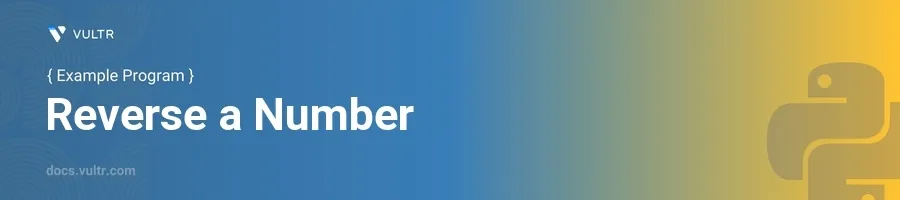
Introduction
Reversing a number in Python is a straightforward yet intriguing operation that is widely used in programming tasks such as algorithms and problem-solving challenges. This technique can help in understanding how to manipulate data at a granular level, which can be critical in areas such as cryptography, optimizations, and during coding interviews.
In this article, you will learn how to reverse a number using various methods in Python. You'll explore this through a series of examples that utilize different Pythonic approaches, such as string slicing, mathematical manipulation, and recursion, enabling you to understand the nuances of each method.
Reversing a Number Using String Slicing
Convert the Number to a String and Reverse
Start by converting the number to a string.
Use string slicing to reverse the string.
Convert the reversed string back to an integer.
pythondef reverse_number(n): reversed_str = str(n)[::-1] return int(reversed_str) # Example usage: number = 12345 reversed_number = reverse_number(number) print(reversed_number)
This code snippet converts a number to a string and uses string slicing (
[::-1]
) to reverse the order of characters. It then casts the reversed string back to an integer. The output fornumber = 12345
will be54321
.
Reversing a Number Using Loops
Perform Mathematical Operations
Initialize a variable to store the reversed number.
Use a while loop to extract each digit from the number and append it to the reversed number's correct position.
Output the reversed number.
pythondef reverse_number_math(n): reversed_num = 0 while n > 0: reversed_num = reversed_num * 10 + n % 10 n //= 10 return reversed_num # Example usage: number = 12345 reversed_number = reverse_number_math(number) print(reversed_number)
In this example, the number is continuously divided by 10, and the remainder (last digit) is added to the reversed number at the progressing tens place. The output is
54321
for the input12345
.
Reversing a Number Using Recursion
Implement Recursive Function to Reverse
Define a recursive function that splits the number and reconstructs it in the reverse order.
Carefully manage the base case and the reduction step to avoid infinite recursion.
Return the reconstructed reversed number.
pythondef reverse_number_recursive(n, reversed_num=0): if n == 0: return reversed_num else: reversed_num = reversed_num * 10 + n % 10 return reverse_number_recursive(n // 10, reversed_num) # Example usage: number = 12345 reversed_number = reverse_number_recursive(number) print(reversed_number)
This recursive function continues to call itself, each time reducing the number by a factor of ten and adjusting the reversed number accordingly. The recursion stops when the number becomes zero, and at this point, the completely reversed number is returned.
Conclusion
Reversing a number in Python can be achieved through multiple approaches, each with its unique style and use case. The string slicing method is the most straightforward for those familiar with Python's string operations. The mathematical approach offers a deeper understanding of number manipulation, while the recursive method demonstrates how to handle more intricate control flows in function calls. By mastering these methods, enhance your ability to manipulate and understand numeric data effectively in your programming projects.
No comments yet.