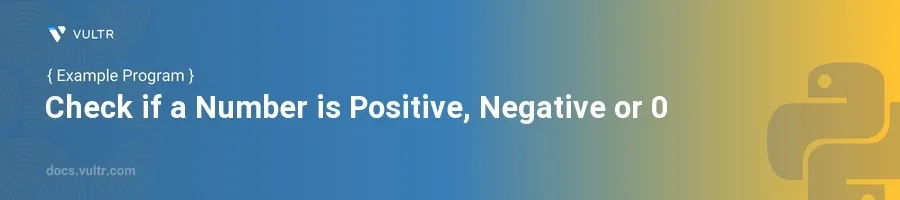
Introduction
Determining whether a number is positive, negative, or zero is a basic but essential operation in many computer programs, particularly in applications dealing with mathematical operations and data analysis. This task involves checking the sign of a given number and returning an appropriate response based on its value.
In this article, you will learn how to create a Python program to check if a number is positive, negative, or zero using simple examples. Implement these methods to enhance basic decision-making capabilities in your Python scripts.
Example of Checking Number Sign
Check a Single Number
Accept input from the user and convert it to an integer.
Use conditional statements to check if the number is positive, negative, or zero.
pythonnum = int(input("Enter a number: ")) if num > 0: print("The number is positive.") elif num < 0: print("The number is negative.") else: print("The number is 0.")
This example asks the user to input a number. It then uses an
if-elif-else
structure to check if the number is greater than, less than, or equal to 0, printing a corresponding message.
Handling Multiple Numbers Using a Function
Define a function that takes a number as an argument and checks its sign.
Call this function with different numbers.
pythondef check_number_sign(num): if num > 0: return "Positive" elif num < 0: return "Negative" else: return "Zero" # Example calls print(check_number_sign(10)) # Positive print(check_number_sign(-5)) # Negative print(check_number_sign(0)) # Zero
This script defines a function
check_number_sign
that returns whether a number is positive, negative, or zero. Different numbers are passed to this function, and it prints out the result. This approach is useful when the sign check needs to be performed multiple times throughout the code.
Using the Function in a Real-world Scenario
Loop through a list of numbers and use the function to check each number's sign.
Print results in a readable format.
pythonnumbers = [7, -3, 0, 12, -9, 0] for number in numbers: sign = check_number_sign(number) print(f"The number {number} is {sign}.")
In this example, a list of numbers is processed by iterating through it with a
for
loop. For each number, thecheck_number_sign
function is called, and the result is printed in a formatted sentence. This demonstrates how to integrate the function into a workflow that requires checking multiple numbers.
Conclusion
The Python examples provided demonstrate straightforward techniques for checking if a number is positive, negative, or zero. By employing conditional statements and functions, you effectively determine the sign of numbers in any given dataset. Utilize these methods in various programming scenarios to simplify and streamline your decision-making processes in Python applications. Ensure your code remains efficient and readable by adopting these structured approaches to basic numerical checks.
No comments yet.