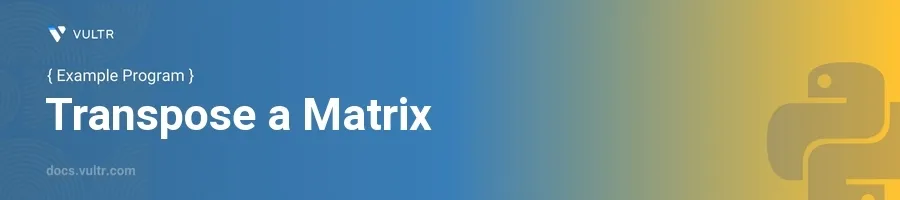
Introduction
Transposing a matrix is a common task in mathematics and programming that involves swapping the matrix's rows with its columns. This operation is crucial in various applications such as computer graphics, solving linear equations, and optimizing algorithms for better performance. Python, with its robust libraries and simple syntax, provides several methods to efficiently transpose a matrix.
In this article, you will learn how to transpose a matrix in Python using multiple approaches. Explore how to accomplish this task using nested list comprehensions, the zip
function, and by leveraging the powerful NumPy library.
Transposing a Matrix with List Comprehension
List comprehensions in Python offer a concise way to create lists. Utilize this feature to transpose a matrix without the need for external libraries.
Example: Basic Matrix Transposition
Define a matrix as a list of lists.
Use nested list comprehension to swap rows and columns.
pythonmatrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] transposed_matrix = [[row[i] for row in matrix] for i in range(len(matrix[0]))] print(transposed_matrix)
This code snippet creates a new matrix
transposed_matrix
where the inner list comprehension[row[i] for row in matrix]
collects thei
-th element from each row, effectively building the columns of the new transposed matrix.
Advantages and Use Cases
Use list comprehensions to transpose matrices when dealing with small to medium-sized matrices and when NumPy is not a dependency in your project.
Using the zip() Function
The built-in zip()
function in Python makes it easy to aggregate elements from multiple iterables. Learn to use zip()
along with list comprehension to transpose matrices.
Example: Transposition Using zip()
Define your matrix as a list of lists.
Apply the
zip()
function with unpacking to transpose the matrix.Convert the result from
zip()
into a list of lists.pythonmatrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] transposed_matrix = list(map(list, zip(*matrix))) print(transposed_matrix)
Using
zip(*matrix)
effectively unzips the rows and recombines them as columns. Themap(list, ...)
is used to ensure that the rows of the transposed matrix remain lists rather than tuples, which is the default output ofzip()
.
Benefits and Practical Applications
Utilize this method for a succinct and readable one-liner solution. It's particularly useful when readability is a priority and performance is not the primary concern.
Employing NumPy for Matrix Transposition
NumPy is a popular library in Python that provides support for large, multi-dimensional arrays and matrices. Discover how to use NumPy for efficient matrix operations, including transposition.
Example: NumPy Transposition
Import the NumPy library.
Create a NumPy array from your matrix.
Use the
.T
attribute to transpose the matrix.pythonimport numpy as np matrix = np.array([ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]) transposed_matrix = matrix.T print(transposed_matrix)
In this example,
matrix.T
returns a new view of the matrix with axes transposed. This operation is highly optimized and does not involve data copying, making it extremely efficient for large data sets.
When to Use NumPy for Transposition
Opt for NumPy when working with large matrices or when already using NumPy arrays in your project for other computations. It's also ideal when performance is critical.
Conclusion
Transposing a matrix in Python can be achieved through various methods, each suitable for different scenarios. Whether opting for a quick solution using zip()
and list comprehension, or a performance-oriented approach with NumPy, Python provides the flexibility to choose according to your project's requirements. By mastering these techniques, you ensure that matrix operations in your applications are efficient and tailored to the context of use.
No comments yet.