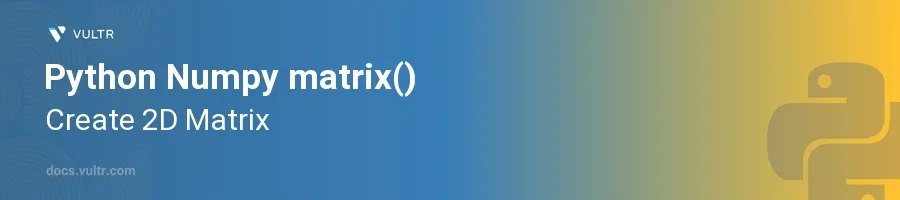
Introduction
The numpy.matrix()
function in Python is a specialized two-dimensional array that offers convenient arithmetic operations mimicking those of classical linear algebra. Known for its powerful handling of matrices, it has been a cornerstone inside the scientific and engineering applications designed with Python. This method is especially tailored for operations involving matrices, providing a rich set of features dedicated to matrix manipulations.
In this article, you will learn how to utilize the numpy.matrix()
function to create 2D matrices efficiently. Explore different types of matrices like identity, filled, and custom data matrices, and understand how to manipulate these using numpy functionalities.
Creating Basic 2D Matrices
Using numpy.matrix() for Direct Matrix Creation
Import the
numpy
library.Use the
matrix()
function with a string input or a list to create a 2D matrix.pythonimport numpy as np # Creating a matrix using a string input mat1 = np.matrix('1 2; 3 4') print(mat1) # Creating a matrix from a list of lists mat2 = np.matrix([[1, 2], [3, 4]]) print(mat2)
Both
mat1
andmat2
produce the same output, a 2D matrix. The string input is convenient for quickly setting up matrices in a compact form.
Generating Special Types of Matrices
Identity Matrix
Use the
numpy.eye()
function to create an identity matrix of specified size.pythonidentity_matrix = np.eye(3) # Creates a 3x3 identity matrix print(identity_matrix)
The identity matrix is crucial in various linear algebra operations and
np.eye()
delivers this matrix instantly.
Zero Matrix
Utilize the
numpy.zeros()
function to create a matrix filled with zeros.pythonzero_matrix = np.zeros((2,3)) # Creates a 2x3 matrix of zeros print(zero_matrix)
A zero matrix is particularly useful as a default or initial-value matrix in many algorithms and transformations.
Manipulating 2D Matrices
Matrix Transposition
Use the
.T
attribute to transpose the matrix.pythontransposed_mat = mat1.T print(transposed_mat)
Transposing is a fundamental operation in matrix manipulation, effectively flipping the matrix over its diagonal.
Matrix Inversion
Apply the
numpy.linalg.inv()
function to invert a matrix.python# Ensure the matrix is square invertible_mat = np.matrix('1 2; 3 4') inverted_mat = np.linalg.inv(invertible_mat) print(inverted_mat)
Matrix inversion is critical in solving systems of linear equations and performing complex transformations.
Conclusion
The numpy.matrix()
method in Python simplifies the process of creating and managing two-dimensional matrices. By exploring different methods to generate various types of matrices and manipulating them using numpy's linear algebra tools, you enhance the effectiveness of your numeric computations and simulations. Employ these practices to make complex matrix operations more manageable and to leverage the full power of numpy in scientific computing.
No comments yet.