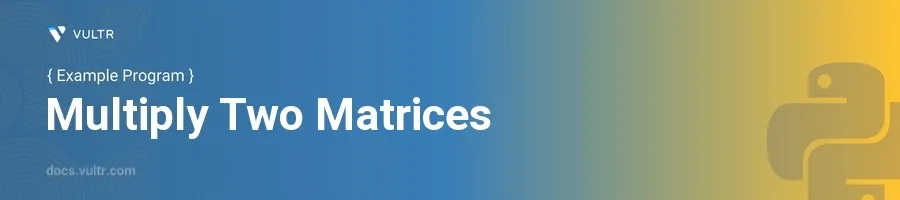
Introduction
Multiplying matrices is a fundamental operation in various applications such as mathematics, physics, engineering, and computer science, particularly in the fields of linear algebra, graphics transformations, and machine learning algorithms. This process involves taking two matrices and producing a third matrix that represents their product, following specific arithmetic rules.
In this article, you learn how to multiply two matrices using Python. This includes setting up the matrices, performing the multiplication, and handling matrices of different sizes. You'll see clear examples to demonstrate each step of the matrix multiplication process using typical programming structures in Python.
Setting Up Your Matrices in Python
Before multiplying two matrices, ensure they conform to the rule of matrix multiplication, which states that the number of columns in the first matrix must be equal to the number of rows in the second matrix. Here is how to set up matrices in Python:
Define two matrices as lists of lists.
pythonA = [[1, 2, 3], [4, 5, 6]] B = [[1, 4], [2, 5], [3, 6]]
Matrix A is a 2x3 matrix (2 rows and 3 columns), and matrix B is a 3x2 matrix (3 rows and 2 columns).
Verify the dimensions to ensure multiplication is possible.
pythonrows_A = len(A) cols_A = len(A[0]) rows_B = len(B) cols_B = len(B[0]) if cols_A != rows_B: print("Cannot multiply the matrices. Incorrect dimensions.")
This step checks if the number of columns in A is equal to the number of rows in B, which is necessary for multiplication to proceed.
Performing Matrix Multiplication
Once matrices are defined and validated, proceed with the multiplication. The product matrix will have a number of rows equal to the number of rows in the first matrix and a number of columns equal to the number of columns in the second matrix.
Initialize the result matrix with zeros.
pythonresult = [[0 for x in range(cols_B)] for y in range(rows_A)]
This sets up a result matrix of the correct size, initially filled with zeros.
Use nested loops to multiply matrices and store the result.
pythonfor i in range(rows_A): for j in range(cols_B): for k in range(cols_A): result[i][j] += A[i][k] * B[k][j]
In these loops,
i
indexes rows of the first matrix,j
indexes columns of the second matrix, andk
iterates over the elements in the row of A and the column of B to compute each component of the product matrix.Print or return the result matrix.
pythonfor r in result: print(r)
This will output the result of the matrix multiplication.
Handling Matrices of Different Sizes
Matrix multiplication is strict about the dimensions of the involved matrices. Here is how to deal with matrices when they do not meet the standard dimensions requirements:
- Ensure proper size condition checks as shown in the setup examples.
- Appropriately manage errors or conditions of incompatible matrices by providing clear messages or handling exceptions in a way that aids debugging or further computation attempts.
Conclusion
Multiplying two matrices in Python requires a good understanding of list handling and loop structuring. By following the examples provided, you enhance your ability to perform complex matrix operations that are crucial in many technical and scientific computing scenarios. Employ these techniques in your projects to efficiently handle mathematical computations involving matrices. With these skills, you ensure robustness and accuracy in your computational tasks involving matrix algebra.
No comments yet.