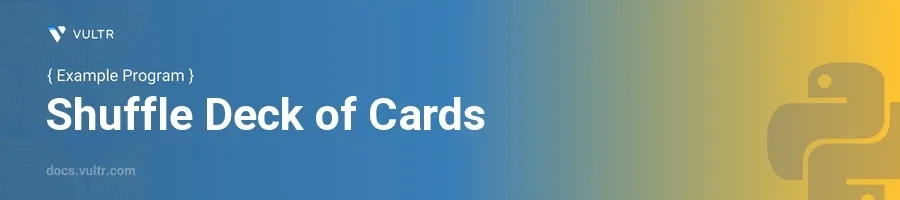
Introduction
Shuffling a deck of cards is a common requirement in games and simulations involving cards. In Python, this task can be efficiently handled with the help of modules like random
which provide methods specifically designed for randomizing the order of items in a list. This emulation of shuffling can be particularly useful in creating algorithms that need to simulate real-world scenarios where randomness is a factor, such as in card games.
In this article, you will learn how to effectively shuffle a deck of cards using Python. Explore code examples that demonstrate how to create a deck, shuffle it, and ensure the randomness, all of which are crucial for applications such as online card games or any statistical simulations involving shuffled decks.
Creating and Shuffling a Deck
Initialize the Deck of Cards
Understand that a standard deck consists of 52 cards comprising four suits: hearts, diamonds, clubs, and spades; each suit contains 13 ranks from 2 to 10, followed by Jack, Queen, King, and Ace.
Use list comprehensions to generate a deck of cards.
pythonsuits = ['Hearts', 'Diamonds', 'Clubs', 'Spades'] ranks = ['2', '3', '4', '5', '6', '7', '8', '9', '10', 'Jack', 'Queen', 'King', 'Ace'] deck = [f"{rank} of {suit}" for suit in suits for rank in ranks] print(deck)
This code generates a list called
deck
, where each card is a combination of a rank and a suit. Upon completion,deck
contains 52 elements, each uniquely representing a card.
Shuffle the Deck
Import the
random
module, which includes functions that support random operations.Use the
shuffle
method from therandom
module to randomize the order of the elements in the deck.pythonimport random random.shuffle(deck) print(deck)
The
shuffle
method rearranges the items in the listdeck
in-place, meaning the order ofdeck
is changed without creating a new list.
Ensuring the Randomness
Testing Shuffle Uniqueness
Recognize the importance of testing the shuffle to ensure it provides a good distribution of randomness, especially in games or simulations.
Execute multiple shuffles and track the order of outcomes.
pythonresults = set() for _ in range(1000): random.shuffle(deck) results.add(tuple(deck)) print(len(results))
By converting the list
deck
to a tuple, you can add it to aset
to track unique shuffles. After numerous shuffles, if the set size approaches the number of trials (which is large), it suggests good randomness.
Advanced Shuffle Techniques
Simulating a Human Shuffle
Consider that human shuffling isn't perfectly random. One might mimic this by cutting the deck a few times during shuffling.
Modify the shuffle process to include a cut operation.
pythondef human_like_shuffle(deck): for _ in range(5): # Shuffle 5 times random.shuffle(deck) cut = random.randint(10, 42) # Assume a cut anywhere between 10 to 42 cards deck = deck[cut:] + deck[:cut] return deck deck = human_like_shuffle(deck) print(deck)
Here, the deck is shuffled multiple times, and a "cut" in different positions simulates a more human-like randomization. The deck is then reassigned to the shuffled and cut version.
Conclusion
Utilizing the Python random
module to shuffle a deck of cards proves to be an efficient approach for card-game programming and other simulations necessitating random modularization. By leveraging Python's capacities, especially through the use of list comprehensions and in-built shuffling methods, you achieve both simplicity and functionality in your programs. Adjust the level of complexity in shuffles to simulate different scenarios, from perfectly random machine shuffles to imperfect human-like shuffles, to better suit your application's requirements.
No comments yet.