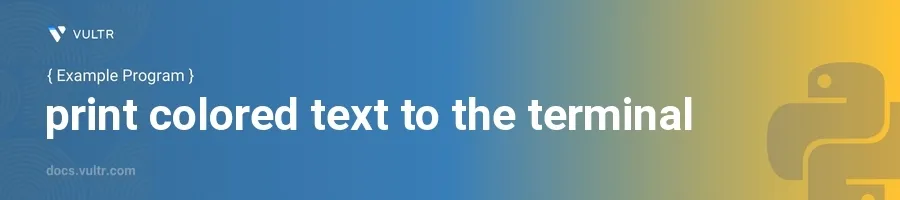
Introduction
Printing colored text to the terminal can significantly enhance the readability of output, making it easier to distinguish between different types of information—such as errors, warnings, and general information. This is particularly useful in debugging scenarios, where critical issues must stand out for immediate attention.
In this article, you will learn how to print colored text to the terminal using Python. By leveraging library support and exploring different methodologies, you can incorporate colored outputs into your Python scripts for more vibrant and distinguishable console logs.
Adding Color Using the termcolor
Module
Install the termcolor
Module
To begin, install the termcolor
module if it's not already available in your Python environment. Use the following command:
pip install termcolor
Basic Text Coloring with termcolor
Import the
colored
function from thetermcolor
module.Apply the
colored
function to the text you wish to print, specifying the desired color as an argument.pythonfrom termcolor import colored print(colored('Hello, World!', 'red'))
This code prints the phrase "Hello, World!" in red in the terminal. The function
colored
requires at least two arguments: the text string and the color name.
Combine Text Styles
Explore combining text styles such as bold, underlined, and background colors.
Extend the
colored
function usage with additional style parameters.pythonprint(colored('Important Warning!', 'yellow', 'on_red', ['bold', 'blink']))
This snippet will display the message "Important Warning!" in bold and blinking yellow text with a red background. The
colored
function takes additional optional arguments for text styles and background color prefixed by 'on_'.
Using ANSI Escape Sequences
Understand ANSI Escape Codes
ANSI escape sequences are a low-level solution for coloring text in terminals that support ANSI colors. These sequences are embedded directly within the text.
Implementing ANSI Escape Codes in Python
Define commonly used ANSI escape sequences for various colors.
Concatenate these sequences with your text to style it.
Reset the styling to default after the text to avoid undesired color leaks in terminal.
pythonRED = '\033[31m' RESET = '\033[0m' print(f'{RED}Error: Failed to load configuration!{RESET}')
This code snippet prints the error message in red. The
RED
variable contains the ANSI escape code for red, and theRESET
restore default formatting.
Tips for Using ANSI Codes
- Remember to reset the formatting to prevent affecting the next lines of output.
- Use ANSI codes for simple color changes when dependencies like
termcolor
are not desired.
Conclusion
Enhance your Python scripts' usability and clarity by printing colored text to the terminal. With the use of the termcolor
module and ANSI escape sequences, you provide clear, visually distinct outputs that can help in efficiently conveying messages, warnings, or errors. This not only aids in debugging but also in creating a more user-friendly command-line interface. Implement these methods in your Python coding practices to bring life and clarity to terminal outputs.
No comments yet.