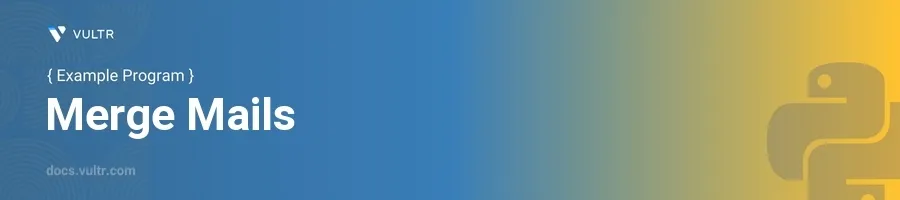
Introduction
Merging mails programmatically is a frequent requirement in scenarios where mass emails need to be customized and sent to a list of recipients. This task involves automating the process of embedding recipient-specific data into a generic email template before sending. Such automation is highly efficient in scenarios like newsletter distributions, event notifications, and personalized marketing campaigns.
In this article, you will learn how to effectively merge mails using Python. Discover how to leverage Python’s string formatting to integrate user data into email templates, and see practical examples that illustrate the merging process. This tutorial ensures you learn to apply these techniques for mailing processes in a way that can be scaled for a large number of recipients.
Preparing the Data for Mailing
Organize Your Recipient Data
Collect all the necessary data for the recipients. Typically, this involves names, email addresses, and any other specific information relevant to the email content.
Store this information in a structured format. For practical purposes in Python, using a list of dictionaries can be quite effective as each dictionary can represent a recipient with name-value pairs for their corresponding data.
pythonrecipients = [ {"name": "Alice", "email": "alice@example.com", "date": "2023-01-01"}, {"name": "Bob", "email": "bob@example.com", "date": "2023-01-02"} ]
This list contains dictionaries where each dictionary includes the name, email, and an example date field which might be relevant for a personalized anniversary email, for example.
Create an Email Template
Define a basic template for the email message. This template will have placeholders which will be filled with recipient data.
Utilize Python’s formatted string literals (f-strings) or the
str.format()
method to embed variables into the placeholders.pythontemplate = """ Dear {name}, This is a reminder about your upcoming event on {date}. We look forward to seeing you soon! Best regards, Your Friendly Team """
This template is structured with placeholders for the recipient's name and a specific date that will be personalized for each recipient.
Merging the Data with the Template
Implement the Merging Function
Iterate over each recipient data in your list.
For each recipient, use the template to create a personalized email content by replacing placeholders with actual data from the dictionary.
Print or store the personalized emails for further use (like sending them).
pythondef merge_mails(recipients, template): merged_emails = [] for recipient in recipients: email_content = template.format(**recipient) merged_emails.append(email_content) return merged_emails personalized_emails = merge_mails(recipients, template) for email in personalized_emails: print(email)
Here, the
merge_mails
function processes each recipient's dictionary by unpacking its values into the template using theformat
function. The resulting listpersonalized_emails
contains all the customized email messages ready to be sent.
Conclusion
Merging mails in Python by leveraging data structures and text formatting provides a robust method for personalizing bulk email delivery. This technique reduces errors, saves time, and enables more effective communication. By structuring recipient data appropriately and utilizing templates with placeholders, you maintain a high level of customization and readability in your emails. Utilize these examples to integrate automated mail merging processes into your applications, ensuring efficient and personalized correspondence with large lists of recipients.
No comments yet.