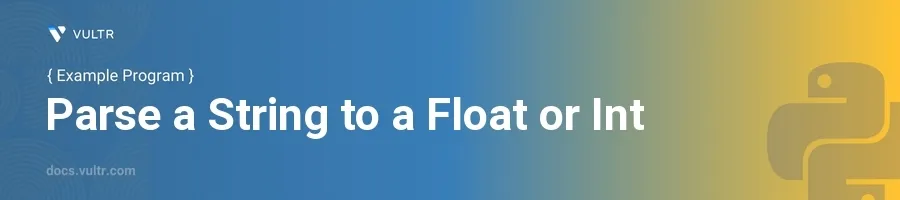
Introduction
Parsing strings into numbers is a critical skill in Python, as it enables the conversion of data received as text (e.g., from user inputs or file readings) into numbers that can be mathematically manipulated. This task comes in handy in scenarios ranging from data preprocessing in machine learning to simple arithmetic operations in daily scripting.
In this article, you will learn how to convert strings into floating-point numbers and integers in Python. By using built-in Python functions and handling common errors, enhance your code's robustness and functionality. Examples will focus on practical scenarios to illustrate how these conversions are performed effectively.
Converting Strings to Integers
When you need to perform mathematical operations on data that comes as strings, converting these strings to integers is essential. Here’s how to do it safely and efficiently:
Basic Conversion
Use the
int()
function to convert a string that represents a whole number into an integer.pythoninteger_value = int("123") print(integer_value)
The function converts the string
"123"
into the integer123
.
Handling Invalid Inputs
Handle cases where the string might not be a valid integer using try-except blocks.
pythontry: integer_value = int("xyz") print(integer_value) except ValueError: print("Invalid input for integer conversion")
This snippet attempts to parse
"xyz"
as an integer, which is not possible, so it catches theValueError
and prints a custom error message.
Converting Strings to Floats
Floating-point numbers are commonly used in computations that require precision, such as measurements and financial calculations. Here’s how you can parse strings into floats in Python:
Basic Float Conversion
Use the
float()
function to convert a string to a floating-point number.pythonfloat_value = float("123.456") print(float_value)
The string
"123.456"
is converted to the floating-point number123.456
.
Handling Locale-Specific Decimal Separators
Adapt your parsing logic to handle locale-specific decimal separators by replacing the separator before conversion.
pythonimport locale locale.setlocale(locale.LC_ALL, 'de_DE') decimal_string = "123,456" normalized_string = decimal_string.replace(',', '.') float_value = float(normalized_string) print(float_value)
In Germany, for example, commas are used as decimal separators. The code changes the comma to a dot to allow for the float conversion.
Advanced Parsing Techniques
When dealing with complex string formats, additional parsing steps might be necessary to extract numeric values successfully.
Extracting Numbers from Mixed Strings
Use regular expressions to extract numbers from strings that contain both text and numbers.
pythonimport re mixed_string = "The temperature is 23.5 degrees Celsius." numbers = re.findall(r"[-+]?[\d]*\.?[\d]+", mixed_string) number = float(numbers[0]) # Assuming we want the first number print(number)
This code uses the
re.findall()
method to find all occurrences of patterns that match numbers. It then converts the first found number into a float.
Conclusion
Mastering string-to-number conversions in Python is pivotal for data manipulation and ensures that your applications can process and analyze data effectively. By utilizing the int()
and float()
functions and preparing for error handling with proper techniques, maintain the integrity and reliability of your data processing systems. Whether part of a large-scale application or simple utility scripts, these skills will significantly enhance your programming prowess in Python. Implementing the techniques discussed ensures your code remains clean, readable, and adaptable to various data inputs.
No comments yet.