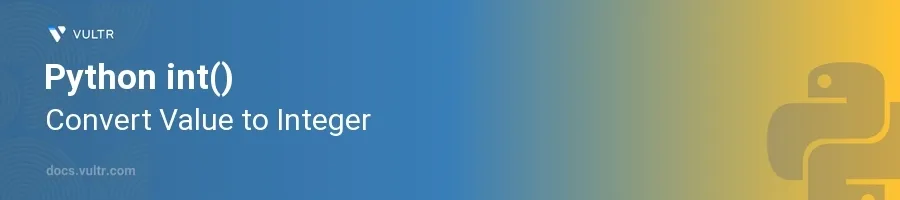
Introduction
The int()
function in Python is a standard function that converts a given input into an integer. This includes parsing integers from different types such as strings or floating-point numbers, especially useful in scenarios where data type conversion is essential, such as user inputs or processing external data files.
In this article, you will learn how to effectively use the int()
function in Python. Discover various ways to convert different data types to integers, handle common exceptions that may arise, and explore ways in which this conversion can be particularly useful in your programming projects.
Understanding the int() Function
Basic Conversion from String to Integer
Take a string that represents a number.
Use the
int()
function to convert this string into an integer.pythonnumber_str = "123" number_int = int(number_str) print(number_int)
This code snippet converts the string
'123'
into the integer123
. Theint()
function achieves this by parsing the number from the string.
Converting Floating-Point Numbers
Start with a floating-point number.
Convert the float to an integer using
int()
.pythonnumber_float = 123.456 number_int = int(number_float) print(number_int)
Here, the
int()
function converts the float123.456
to the integer123
. This function truncates the decimal part and retains only the whole number.
Handling Non-numeric Strings
Attempt to convert a non-numeric string to an integer to observe the behavior.
Catch the
ValueError
exception to handle this error gracefully.pythonnon_numeric = "abc" try: result = int(non_numeric) except ValueError: print("Cannot convert non-numeric string to integer")
This example shows how using
int()
on a non-numeric string raises aValueError
. The exception handling prevents the program from crashing and provides a user-friendly message instead.
int() with Base Specifications
Convert Binary String to Integer
Have a binary string like '1010'.
Use
int()
with the base parameter set to 2 to convert this to a decimal integer.pythonbinary_str = "1010" decimal_int = int(binary_str, 2) print(decimal_int)
This converts the binary string
'1010'
to the decimal integer10
. The second parameter inint()
specifies that the number is in base 2.
Convert Hexadecimal String to Integer
Start with a hexadecimal string such as '1a'.
Convert it to an integer using
int()
with the base set to 16.pythonhex_str = "1a" decimal_int = int(hex_str, 16) print(decimal_int)
The hexadecimal string
'1a'
is converted to the integer26
using base 16.
Conclusion
The int()
function in Python offers a versatile approach for converting various data types to integers, which is crucial in numerous programming contexts where type consistency is important. Whether handling user input, processing text files, or working with binary and hexadecimal data, leveraging the int()
function ensures that your data is properly typecast, supporting robust and error-free code. By mastering int()
, you enhance your capacity to build flexible and reliable Python applications.
No comments yet.