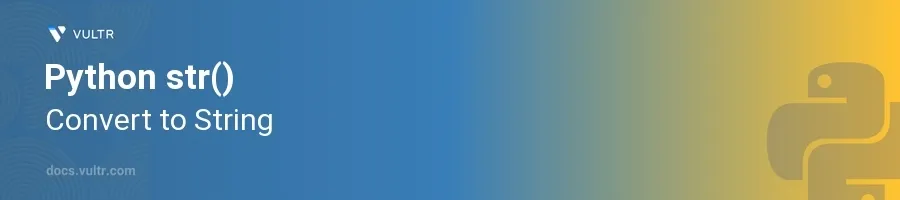
Introduction
The str()
function in Python is essential for converting values into their string representation. This function is versatile, being applicable to a vast range of data types including integers, floats, and complex objects. Utilizing str()
effectively allows for easier data manipulation, logging, and user-friendly display outputs in applications.
In this article, you will learn how to employ the str()
function across various data types. Discover how to transform non-string data into strings and explore the impact of this transformation on data handling and presentation.
Using str() with Basic Data Types
Convert Integers to Strings
Define an integer.
Convert the integer into a string using
str()
.pythonnumber = 123 string_number = str(number) print(string_number) print(type(string_number))
Here,
str()
is applied to the integernumber
, converting it into the stringstring_number
. Thetype()
function confirms that the conversion results in a string type.
Convert Floating Point Numbers to Strings
Initiate a floating point number.
Apply the
str()
function to convert it to a string.pythonfloat_number = 98.6 string_float = str(float_number) print(string_float) print(type(string_float))
In this example, the floating point
float_number
is converted to a stringstring_float
usingstr()
. Usingtype()
, verify that the returned object is indeed a string.
Handling Booleans
Start with a boolean value.
Use
str()
to transform the boolean into a string.pythonboolean_value = True string_boolean = str(boolean_value) print(string_boolean) print(type(string_boolean))
This code block converts the boolean
boolean_value
to a stringstring_boolean
throughstr()
. The outcome is checked withtype()
, confirming the transformation.
Transforming Complex Objects into Strings
Converting Lists to Strings
Create a list of multiple items.
Convert the entire list into a string with
str()
.pythonmy_list = [1, 2, 3, 'hello'] list_as_string = str(my_list) print(list_as_string) print(type(list_as_string))
This snippet turns the list
my_list
into a stringlist_as_string
. Examining it withtype()
confirms it's now a string, capturing the entire list structure.
Convert Dictionaries to Strings
Define a dictionary containing various data types.
Use the
str()
function to convert the dictionary to its string representation.pythonmy_dict = {'id': 1, 'name': 'John', 'active': True} dict_as_string = str(my_dict) print(dict_as_string) print(type(dict_as_string))
By applying
str()
tomy_dict
, the whole dictionary is transformed into the stringdict_as_string
. Verification usingtype()
shows that the result is a string type.
Conclusion
The str()
function in Python provides a simple yet powerful means of converting various data types into strings. Employing this function can dramatically simplify the process of data serialization, logging, and user-interface development by ensuring that all data fields are in a readable and manageable string format. By mastering the use of str()
demonstrated through the examples provided, you can ensure seamless management and display of data across different sections of your applications.
No comments yet.