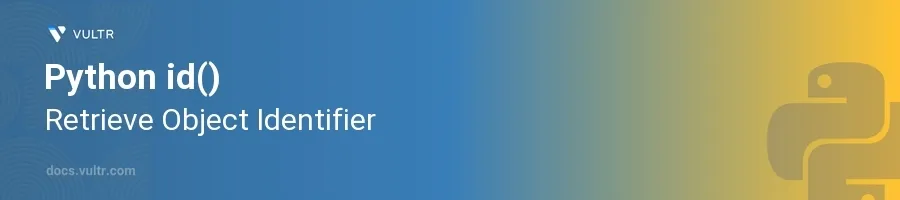
Introduction
The id()
function in Python provides a unique identifier for any object during its lifetime in a Python session. This identifier is a memory address in CPython, which is the most widely used Python implementation. Understanding how to utilize id()
is crucial for debugging and comparing objects at a low level.
In this article, you will learn how to effectively use the id()
function to retrieve identifiers for different types of objects in Python. Discover the nuances of object identity and the implications of these identities in your Python code.
Utilizing the id() Function
Retrieve Identifier of Simple Data Types
Create simple data types like integers, strings, or booleans.
Use the
id()
function to fetch their memory addresses.pythonx = 10 y = "Hello" z = True print(id(x)) print(id(y)) print(id(z))
The above snippet prints the unique identifier for each object, which represents its memory address in CPython. This helps in understanding how Python manages memory for simple data types.
Understanding Identifiers with Immutable Types
Explore how identifiers behave with immutable types like tuples.
Generate multiple instances and compare their identifiers.
pythontuple1 = (1, 2, 3) tuple2 = (1, 2, 3) print(id(tuple1)) print(id(tuple2))
Here,
tuple1
andtuple2
are distinct objects, hence they have different identifiers. This illustrates that even identical immutable objects have separate identities unless explicitly made identical.
Observing Identifier Reuse
Note that Python might reuse identifiers for small integers and interned strings due to optimization techniques.
Create variables, delete them, and recreate to see if ids are reused.
pythona = 256 print(id(a)) del a b = 256 print(id(b))
In this example, both
a
andb
may have the same identifier, indicating Python’s optimization mechanism that reuses object identifiers for small integers.
Managing Identifiers with Mutable Types
Experiment with Lists and Dictionaries
Mutable types like lists and dictionaries maintain consistent identifiers even if their content changes.
Manipulate these types and observe the stability of their identifiers.
pythonlist1 = [1, 2, 3] print(id(list1)) list1.append(4) print(id(list1))
The
id()
value oflist1
remains constant despite the change in its content. This property is crucial for tracking objects throughout their lifecycle in applications.
Implications for Object Comparisons
Use
id()
in logic where identity is more relevant than equality.Demonstrate comparing two lists by identifiers.
pythonlist_a = [5, 6] list_b = list_a print(id(list_a) == id(list_b))
This code snippet shows that
list_a
andlist_b
are the same object because they share the same identifier, useful in scenarios where you need to ensure object uniqueness rather than content equality.
Conclusion
The id()
function in Python is a fundamental tool for understanding and controlling object identity. It serves as a debugging aid and a way to enforce object uniqueness in memory-intensive applications. By applying the strategies discussed, you can handle Python objects more effectively, ensuring your programs are robust and efficient in managing memory and identity checks.
No comments yet.