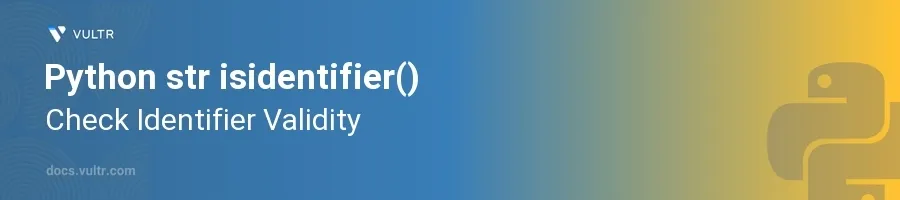
Introduction
The str.isidentifier()
method in Python provides a simple way to determine if a string qualifies as a valid identifier according to Python's language rules. This method is particularly useful when dynamically generating variable names or ensuring that input names comply with Python's naming conventions.
In this article, you will learn how to use the str.isidentifier()
method effectively. Explore its practical applications in scripting and data validation scenarios, and understand how it can help maintain the integrity of your code by verifying identifiers programmatically.
Understanding isidentifier()
What Constitutes a Valid Identifier
- Review Python's rules for valid identifiers:
- They must start with a letter (A-Z, a-z) or an underscore (_).
- The subsequent characters can be letters, underscores, or digits (0-9).
- They cannot be reserved words in Python (like
for
,if
,else
, etc.).
Basic Usage of isidentifier()
Define a string that you suspect might be a valid identifier.
Call the
isidentifier()
method on this string.pythonpotential_identifier = "variable1" print(potential_identifier.isidentifier())
This code checks if the string
variable1
is a valid identifier, which in this case, returnsTrue
.
Edge Cases
Test strings that include special characters.
Assess strings that start with numbers.
Evaluate Python's reserved keywords.
pythonspecial_char = "var!able" starts_with_number = "1variable" reserved_keyword = "class" print(special_char.isidentifier()) # False print(starts_with_number.isidentifier()) # False print(reserved_keyword.isidentifier()) # True, but it's a reserved keyword
Here, the method returns
False
for names that start with numbers or have special characters, andTrue
for reserved keywords. Be aware that while reserved keywords returnTrue
, they cannot be used as identifiers in your code.
Practical Applications
Dynamically Generating Variable Names
Consider a scenario where variable names are generated based on user input.
Ensure that these names are valid identifiers to avoid runtime errors.
pythonuser_input = "123abc" if user_input.isidentifier(): print(f"{user_input} is a valid identifier.") else: print(f"{user_input} is not a valid identifier.")
This snippet helps in validating variable names provided by user inputs, assisting in maintaining robust and error-free code.
Validating Function Names in a Script
When writing a tool that accepts function names as input, validate them using
isidentifier()
.Provide feedback to the user if the input is not a valid identifier.
pythonfunction_name = input("Enter the function name: ") if function_name.isidentifier(): print("Valid function name.") else: print("Invalid function name.")
This ensures that all function names entered in your script meet Python’s identifier naming rules, thereby reducing the risk of syntax errors.
Conclusion
The str.isidentifier()
method in Python is a vital tool for ensuring that strings conform to the rules for identifiers in Python's syntax. It provides a reliable way to programmatically check the validity of names for variables, functions, and other identifiers. By integrating this method into your validation processes, you safeguard your code from potential errors associated with invalid naming conventions. Harness this function effectively to enhance both the robustness and the reliability of your Python scripts.
No comments yet.