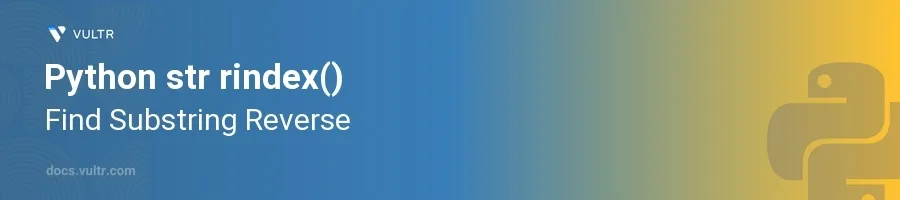
Introduction
The rindex()
method in Python’s string class can be incredibly useful for finding the last occurrence of a substring within another string. This method is particularly helpful when you need to determine the position of a substring from the end of the string towards the beginning, essentially conducting a reverse search.
In this article, you will learn how to utilize the rindex()
method effectively. By examining various practical examples, you'll grasp how to apply this method in real-world programming scenarios to handle substring searches efficiently.
Understanding the rindex() Method
Basic Usage of rindex()
Start by defining a string where you’ll search for a substring.
Use the
rindex()
method by passing the substring as an argument.pythonfull_string = "hello world, hello Python" position = full_string.rindex("hello") print(position)
This code searches for the last occurrence of the substring
"hello"
. It finds it starting at position 13, which is the beginning of the second"hello"
infull_string
.
Handling Exceptions with rindex()
Be aware that
rindex()
will raise aValueError
if the substring is not found.Implement error handling using a
try-except
block to manage this exception gracefully.pythontry: result = full_string.rindex("Java") except ValueError: result = "Substring not found" print(result)
Here, attempting to find
"Java"
infull_string
results in aValueError
because"Java"
does not exist in the string. The exception is caught, and the message"Substring not found"
is printed.
Specifying Start and End Parameters
Recognize that you can limit the search within a specified range using start and end parameters.
Use the start and end parameters to define the search boundaries.
pythonposition_within_bounds = full_string.rindex("hello", 0, 15) print(position_within_bounds)
The
rindex()
function here searches for the substring"hello"
within the first 15 characters offull_string
. It successfully finds it at position 0.
Using rindex() in Real-world Scenarios
Parsing File Paths
Use
rindex()
to find the last directory separator in a filepath, which is common in file handling tasks.Extract the filename from the filepath after the last directory separator.
pythonfilepath = "/home/user/docs/report.txt" last_slash_index = filepath.rindex("/") filename = filepath[last_slash_index + 1:] print(filename)
This snippet retrieves the filename
"report.txt"
by finding the last occurrence of"/"
and slicing the string right after that position.
Data Cleaning
In data processing, use
rindex()
to remove unwanted parts of strings from the end.Identify and strip unwanted characters or substrings from data entries effectively.
pythondata_entry = "error: 404 Page Not Found /error" clean_entry_index = data_entry.rindex("/") clean_entry = data_entry[:clean_entry_index] print(clean_entry)
The code locates the last slash and slices off everything after it to clean the data entry to
"error: 404 Page Not Found"
.
Conclusion
The rindex()
method is a potent tool for string manipulation in Python, providing straightforward solutions for finding the last occurrence of a substring. Understanding how to employ rindex()
effectively enhances your capability to manipulate and process strings in various applications, from file handling to data cleaning. By mastering the examples provided, you bolster your string handling skills and can integrate these techniques into your Python projects to achieve more efficient and cleaner code.
No comments yet.