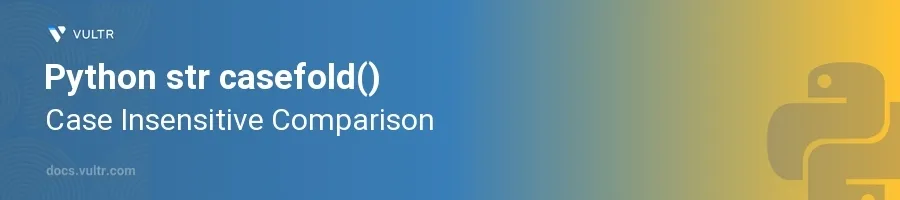
Introduction
The str.casefold()
method in Python is essential for performing case-insensitive text comparisons or lookups. This method is particularly useful when comparing text strings where case variation is irrelevant, such as usernames or hashtags, making it a staple in data preprocessing for natural language processing (NLP) and other text-related tasks.
In this article, you will learn how to effectively utilize the casefold()
method to handle text data in various scenarios. Explore practical examples where case insensitivity is crucial and see how this method can be integrated into text comparison operations to ensure your code handles different textual inputs smoothly.
Understanding the casefold() method
What is casefold()?
- Recognize that
casefold()
is a string method specifically designed for aggressive lowercasing of strings. It goes beyond the basiclower()
method by handling cases influenced by global languages more effectively. - Understand that this method removes all case distinctions present in a string. It is intended for caseless matching, which makes it a more robust option for international applications than traditional lowercasing.
Basic Usage of casefold()
Create a basic string containing mixed case letters.
Apply the
casefold()
method.pythontext = "Hello, World!" print(text.casefold())
Here, the string
text
is transformed into all lowercase letters,"hello, world!"
, throughcasefold()
. This transformation assists in case insensitive comparisons.
Scenario: Comparing Usernames in a User-friendly Manner
Implementing Case Insensitive Checks
Consider a scenario where you are managing user registrations for a website, and usernames are case insensitive.
Prepare to compare input usernames using
casefold()
to ensure that username 'JohnDoe' and 'johndoe' are treated as the same username.pythonusername_db = ["JohnDoe", "AliceInWonderland", "charlie09"] new_username = "johndoe" if new_username.casefold() in map(str.casefold, username_db): print("Username already taken.") else: print("Username is available.")
This code snippet creates a list of current usernames and applies
casefold()
to both thenew_username
and all elements inusername_db
. As a result, it ensures that 'JohnDoe' and 'johndoe' are recognized as the same name, disallowing duplicate usernames regardless of their case.
Handling Multilingual Case Sensitivity
Case Insensitivity in Non-English Characters
Acknowledge that non-English characters can also have case variations that aren’t accounted for by simple lowercasing methods.
Use
casefold()
for robust international text handling.pythongerman_text = "Straße" search_text = "STRASSE" if search_text.casefold() == german_text.casefold(): print("The texts are the same.") else: print("The texts do not match.")
In this example, the German word "Straße" ("street" in English, which also has a case-sensitive variation spelled 'STRASSE') accurately matches the uppercased input 'STRASSE' through
casefold()
. This behavior demonstratescasefold()
's effectiveness across languages and character sets.
Conclusion
The str.casefold()
method in Python is highly valuable for conducting thorough, case-insensitive text comparisons, particularly when dealing with user inputs and multilingual datasets. By implementing this method, you avoid common pitfalls associated with simple case conversion and ensure your text data is handled in a uniform, user- and language-friendly manner. Employ casefold()
in your future text processing tasks to maintain consistency and data integrity across diverse textual inputs.
No comments yet.