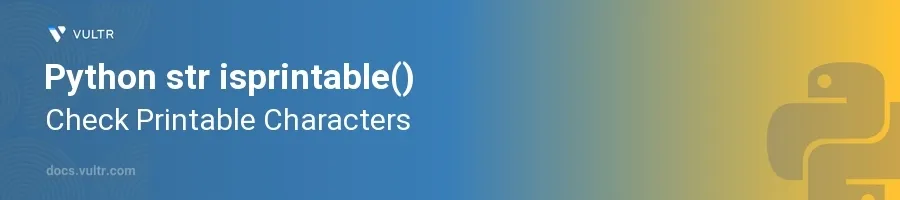
Introduction
The str.isprintable()
method in Python is a straightforward yet powerful string method used to determine if all characters in a string are printable. A "printable" character is one that occupies a printing position on the output (e.g., letter and numerals, punctuation marks, space). This method often comes in handy when validating inputs and ensuring text outputs can be neatly displayed or logged without causing errors due to non-printable characters.
In this article, you will learn how to effectively use the str.isprintable()
method in different scenarios. Master the ability to identify strings that contain only printable characters, and learn how to handle situations where non-printable characters might affect your program's functionality or data processing tasks.
Exploring str.isprintable()
Basic Usage of isprintable()
Start by defining a simple string that you know contains only printable characters.
Call the
isprintable()
method on this string.pythontext = "Hello, World! 123" print(text.isprintable())
This code sample checks whether all characters in the string
text
are printable. As expected, the output will beTrue
because all characters, including letters, comma, space, and numbers, are printable.
Recognizing Non-Printable Characters
Define a string that includes a non-printable character, such as the newline character (
\n
) or tab character (\t
).Use the
isprintable()
method to check the string.pythontext = "Hello, World!\n" print(text.isprintable())
Here, the string
text
includes a newline character, which is not considered printable. The method therefore returnsFalse
.
Mixed Content in Strings
Create a string with a mix of printable and non-printable characters.
Apply the
isprintable()
method to see the output.pythontext = "Data 123\t456\n789" print(text.isprintable())
Despite having mostly printable characters, the inclusion of the tab (
\t
) and newline character (\n
) makes this string non-printable as a whole, soisprintable()
returnsFalse
.
Advanced Use of isprintable()
Filtering Printable from Non-Printable Strings
Generate a list of strings, some entirely printable, others not.
Use a list comprehension combined with
isprintable()
to filter the list.pythontexts = ["Hello!", "Good\nMorning", "Welcome\tHome", "Evening :)", "Have a good day."] printable_texts = [text for text in texts if text.isprintable()] print(printable_texts)
This filters out "Good\nMorning" and "Welcome\tHome" due to their non-printable characters, leaving only completely printable strings in
printable_texts
.
Incorporating isprintable() in Data Validation
Simulate a scenario where user input is validated for being printable.
Trigger different responses based on whether the input is printable or not.
pythonuser_input = input("Enter some text: ") if user_input.isprintable(): print("Thank you for your input!") else: print("Error: Non-printable characters detected.")
This conditional logic ensures that the program only accepts inputs that consist entirely of printable characters, making it robust against certain types of input corruption or formatting errors, which can be crucial for file writing or logging operations.
Conclusion
Utilizing the str.isprintable()
method in Python effectively ensures that strings being processed or displayed contain only characters that are safe and expected to be shown on most outputs. This is especially vital in domains involving data input validation, logging, and any situation where readability and display integrity are of concern. With the examples provided, embed this method in various scenarios to maintain high quality and predictable outputs in your Python applications.
No comments yet.