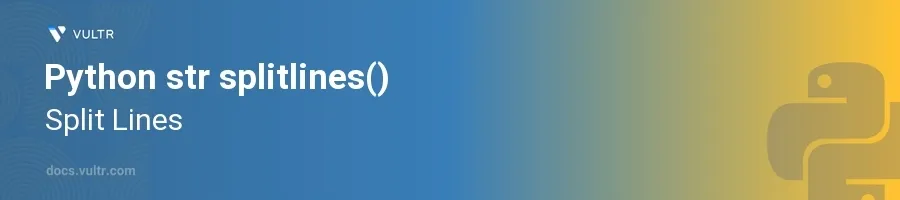
Introduction
The splitlines()
method in Python is an essential tool for dividing a string into a list where each element is a line. This method provides a way of handling multiline strings efficiently, especially when parsing data that contains line breaks. It's particularly useful in applications involving text processing, file reading, or even receiving streamed data that needs splitting into manageable parts.
In this article, you will learn how to effectively use the splitlines()
method across different scenarios. Master how to split strings into lines with or without retaining line break characters, and explore practical applications of this function with various types of multiline strings.
Basics of str.splitlines()
Understanding the Method Syntax
Recognize that the
splitlines()
method is straightforward to use with minimal parameters.Learn the basic form of the method:
pythontext = "Hello\nWorld\nWelcome" lines = text.splitlines() print(lines)
This code splits the string
text
into lines at each newline character and returns the list['Hello', 'World', 'Welcome']
.
Options in splitlines()
Learn about the optional
keepends
parameter.Utilize
keepends
to retain line breaks in the output.pythontext = "Hello\nWorld\nWelcome" lines = text.splitlines(keepends=True) print(lines)
With
keepends=True
, the output includes the line break characters, resulting in['Hello\n', 'World\n', 'Welcome']
.
Practical Applications
Parsing Multiline User Inputs
Handle multiline inputs from users that might include natural line breaks.
Use
splitlines()
to convert the input into a list of lines for easier processing.pythonuser_input = "Name: John Doe\nAge: 30\nLocation: Earth" details = user_input.splitlines() for detail in details: print(detail)
This snippet effectively breaks down user input into separate lines, making it easier to parse and process each detail.
Working with File Content
Read a file that contains multiple lines.
Apply
splitlines()
to manage and manipulate each line separately.pythonwith open('sample.txt', 'r') as file: content = file.read() lines = content.splitlines() for line in lines: print(line)
Here, the file's content is read all at once and split into lines. Each line is then processed individually, simplifying tasks like data parsing or cleanup.
Handling Network Data
Process data received from network transmissions that might be segmented by newline characters.
Split the received data into lines to simulate the receiving end's data processing mechanism.
pythonnetwork_data = "GET / HTTP/1.1\nHost: example.com" request_lines = network_data.splitlines() for line in request_lines: print(line)
The method shown is especially useful in server-side scripting where HTTP requests need to be parsed line-by-line to interpret headers and commands.
Conclusion
The splitlines()
function in Python is a versatile tool for splitting strings into lines. Its ease of use and optional parameters make it adaptable to both simple and complex text processing tasks. By splitting multiline strings efficiently, you enhance the management and manipulation of textual data in your Python programs. Whether you're dealing with user inputs, file contents, or network data, splitlines()
helps ensure your code remains clean, readable, and effective. Apply the strategies discussed to streamline your text processing workflows and achieve robust data handling in your applications.
No comments yet.