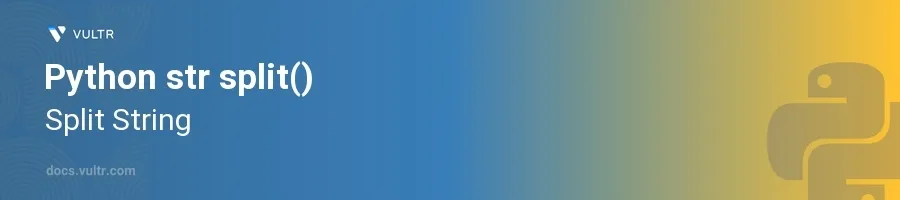
Introduction
The split()
method in Python is an essential tool for dividing a string into a list of substrates based on a specified delimiter. This method is particularly useful when processing textual data, parsing files, or managing user input where string elements need to be individually analyzed or manipulated. It simplifies tasks that involve the separation of components from a string, such as words from sentences or data points from CSV entries.
In this article, you will learn how to effectively utilize the split()
method in various scenarios. Discover different ways to apply this method, understand how it interacts with different types of delimiters, and explore handling cases with multiple consecutive separators.
Basic Usage of str split()
Splitting by Space
Start with a basic string splitted by space.
Use
split()
without any arguments, which defaults to splitting the string by whitespace.pythontext = "Hello world this is Python" result = text.split() print(result)
Here,
split()
divides the stringtext
into words using spaces as the default separator. The result is a list of the individual words:['Hello', 'world', 'this', 'is', 'Python']
.
Custom Delimiter Splitting
Define a string that contains a specific separator.
Specify this separator in the
split()
method to break the string accordingly.pythondata = "apple,orange,banana,grape" fruits = data.split(',') print(fruits)
In this example, the string
data
is split at each comma, resulting in a list of fruit names. The output confirms the list:['apple', 'orange', 'banana', 'grape']
.
Advanced Splitting Scenarios
Handling Consecutive Separators
Deal with inputs where multiple consecutive separators are present.
Understand how
split()
handles these cases by default, splitting and ignoring empty strings between separators.pythonmessy_data = "one;;;two;three;;four" clean_data = messy_data.split(';') print(clean_data)
Notice how
clean_data
includes empty strings corresponding to consecutive semicolons. The output lists each substring, including empty ones where no characters exist between delimiters.
Limiting the Number of Splits
Use the
split()
method with themaxsplit
argument to limit the number of splits.Analyze how the string is divided based on the
maxsplit
value.pythonsentence = "Welcome to Python programming" limited_split = sentence.split(' ', 2) print(limited_split)
By specifying
2
formaxsplit
, the method splits the string only twice, resulting in three parts:['Welcome', 'to', 'Python programming']
. This technique is useful for separating only the initial segments of a string.
Special Cases and Considerations
Splitting with Special Characters
Handle strings containing special characters or escape sequences, like newlines and tabs.
Use these characters as delimiters for splitting.
pythontext_with_newlines = "Line1\nLine2\nLine3" lines = text_with_newlines.split('\n') print(lines)
The output will list each line as a separate element from a string where line breaks act as delimiters:
['Line1', 'Line2', 'Line3']
.
Empty String Handling
Understand the behavior of
split()
when applied to an empty string.See how Python handles this special case.
pythonempty_string = "" split_empty = empty_string.split() print(split_empty)
When
split()
is called on an empty string, it results in an empty list. This is useful for ensuring that operations on empty input do not produce unwanted errors or exceptions.
Conclusion
The split()
function in Python is a versatile tool for breaking down strings into manageable parts. Whether dealing with data extraction, user input parsing, or simply dividing a sentence into words, split()
provides a robust solution. By mastering its usage across various scenarios, including handling special characters and limiting splits, you optimize text processing tasks, making your code more efficient and your data easier to handle. Harness these techniques to improve data manipulation and to enhance overall functionality in your Python scripts.
No comments yet.