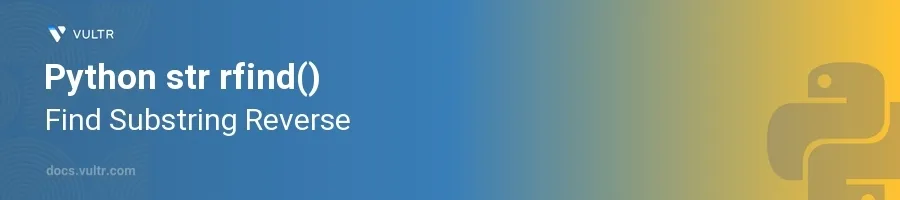
Introduction
The str.rfind()
method in Python is an invaluable tool for searching for substrings within a string from the end, making it ideal for instances where you need to find the position of the last occurrence of a specific character or sequence of characters. Unlike the find()
method, which starts searching from the beginning, rfind()
starts from the right (end) and moves towards the left (start), providing the index of the last match.
In this article, you will learn how to harness the power of str.rfind()
to enhance your string handling operations in Python. Explore practical examples where rfind()
proves to be immensely useful, from basic substring searching to more advanced scenarios involving conditions and slicing.
Understanding str.rfind()
Basic Usage of str.rfind()
Recognize that
str.rfind(sub, start, end)
searches for the substringsub
within an optional range specified bystart
andend
.Get familiar with the function returning
-1
if the substring is not found.pythontext = "Hello, welcome to the Python tutorial." result = text.rfind("Python") print(result)
In this example,
rfind()
finds the substring "Python" starting its search from the end of the string. It returns the index 21, indicating the starting position of "Python" from the beginning.
Handling When Substring is Not Found
Use
str.rfind()
to handle cases where the substring might not be present in the string.Check if the result is
-1
to confidently manage the absence of the substring.pythonsentence = "Example without the specified substring." find_result = sentence.rfind("missing") if find_result == -1: print("Substring not found.")
Here,
rfind()
is asked to find "missing" in the sentence. Since the substring does not exist, the method returns-1
, triggering the print statement to indicate that the substring was not found.
Specifying Search Range
Take advantage of the optional
start
andend
parameters to limit the search within a specific segment of the string.Utilize this feature for efficient searching in large texts or specific data parsing tasks.
pythonnarrative = "Look over there. That is where the treasure lies. Over there, yes there!" position = narrative.rfind("there", 0, 50) print("Last 'there' within first 50 characters: Position", position)
In this case,
rfind()
looks for the last occurrence of "there" within the first 50 characters of the string. Despite multiple occurrences in the complete text, it restricts the search effectively based on the provided range.
Advanced Scenarios
Case Insensitive Search
Convert both the main string and the substring to the same case to perform a case insensitive search.
Implement this method when the case of the text is variable or unknown.
pythonphrase = "This is a Demo string with mixed CASE." query = "demo" case_insensitive_position = phrase.lower().rfind(query.lower()) print(case_insensitive_position)
Here, converting both
phrase
andquery
to lower case before performing therfind()
ensures the position of "demo" is found regardless of its original casing inphrase
.
Conclusion
Mastering the str.rfind()
method in Python opens up a host of possibilities for effectively managing and searching strings. Whether you're dealing with data parsing, validation, or simply trying to extract and process information from strings, rfind()
offers a robust solution. Equip yourself with this method to enhance how you handle strings, making your applications more efficient and your code cleaner. With the techniques discussed, ensure that your programming toolkit is well-rounded and ready for a variety of string manipulation tasks.
No comments yet.