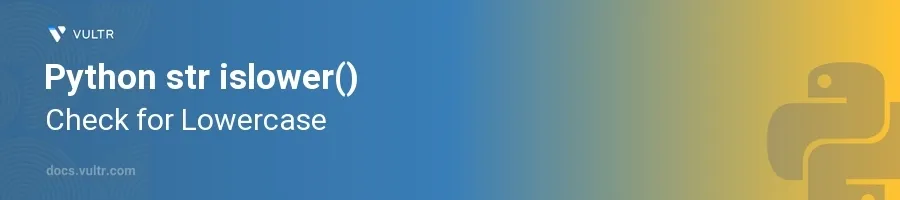
Introduction
The islower()
method in Python is a convenient string method used to determine if all the alphabetic characters in a string are lowercase. This method is particularly useful for data validation, text processing, and conditioning flows based on text format.
In this article, you will learn how to apply the islower()
method in various scenarios to enhance your Python programming skills. Explore how this method can be integrated into different functions and how it behaves with various types of strings, contributing to more robust and reliable code.
Understanding the str islower() Method
Basic Usage of islower()
Use a simple string to check if all characters are in lowercase.
Apply the
islower()
method and print the result.pythontext = "hello world" result = text.islower() print("All lowercase:", result)
This code evaluates the string
text
to check if all alphabetic characters are lowercase. Sincehello world
is entirely in lowercase,islower()
returnsTrue
.
Considerations with Non-Alphabetic Characters
Understand that non-alphabetic characters do not affect the outcome of
islower()
.Use a string that includes digits, spaces, and punctuation to demonstrate this point.
Apply the
islower()
function and print the output.pythontest_string = "example 123!" result = test_string.islower() print("Effect of non-alphabetic characters:", result)
Here, the method checks only the alphabetic characters in
test_string
. Non-alphabetic characters like123!
do not influence the result, and since all alphabetic characters are lowercase, the method returnsTrue
.
Interaction with Empty Strings
Recognize that an empty string will always return
False
whenislower()
is called.Test with an empty string to verify this behavior.
Show the result.
pythonempty_text = "" result = empty_text.islower() print("Empty string:", result)
In this example, since there are no characters to evaluate,
islower()
returnsFalse
for the empty string.
Advanced Applications of islower()
Validating User Input
Use
islower()
to ensure that user inputs adhere to certain conditions, such as being fully lowercase.Simulate a user input scenario where
islower()
can be used for validation.pythonuser_input = input("Enter lowercase text: ") if user_input.islower(): print("Valid Input!") else: print("Invalid Input: Please enter all lowercase letters.")
This piece of code prompts the user to enter text and validates whether it is entirely in lowercase using
islower()
. It provides immediate feedback based on the input's case sensitivity.
Integrating with Other String Methods
Combine
islower()
with other string methods for comprehensive string manipulations and checks.Create a sample code snippet that uses
islower()
alongsidestrip()
to remove leading and trailing spaces before performing the lowercase check.pythontext = " mixed case " cleaned_text = text.strip() result = cleaned_text.islower() print("After stripping spaces:", result)
Here,
strip()
is used first to remove extra spaces from the string. After that,islower()
checks whether the remaining string is in lowercase. This approach is common in data cleaning tasks where input format needs standardization.
Conclusion
The islower()
function in Python is a powerful, straightforward tool for string case evaluation. Mastering its usage ensures that you can effectively handle case sensitivity issues in text processing tasks. From simple data validation to complex input sanitization, incorporating this method enhances the functionality and reliability of your applications. Apply the concepts discussed to tackle common programming challenges involving text and build more intuitive, user-friendly Python applications.
No comments yet.