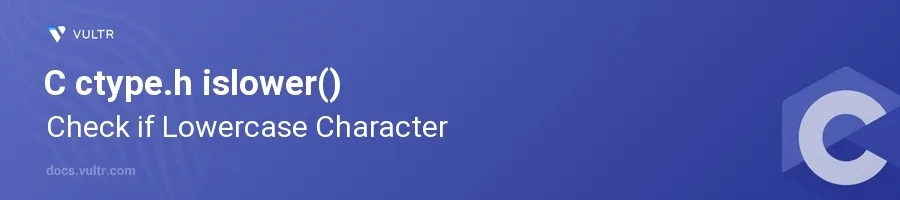
Introduction
The islower()
function from the C standard library's <ctype.h>
header file is a straightforward yet indispensable tool for checking if a character is lowercase. This utility function simplifies the process of validating character casing, which is particularly useful in text processing where case-sensitivity is key.
In this article, you will learn how to use the islower()
function effectively. Understand how this function evaluates characters and implement it to enhance operations that require case condition checks in your C programs.
Understanding islower()
Basic Usage of islower()
Include the
<ctype.h>
header in your C program. This header contains the prototype forislower()
.Pass a character to
islower()
and check its return value.c#include <ctype.h> #include <stdio.h> int main() { char ch = 'a'; if (islower(ch)) { printf("%c is a lowercase letter.\n", ch); } else { printf("%c is not a lowercase letter.\n", ch); } return 0; }
This code demonstrates how to check if the character
ch
is lowercase. Sincech
is 'a',islower()
returns a non-zero value, indicating true, and the message confirms that the character is lowercase.
Evaluating Different Inputs
Experiment with
islower()
by testing various character types.Distinguish between lowercase alphabetic characters and other types.
c#include <ctype.h> #include <stdio.h> int main() { char chars[] = {'a', 'B', '3', '@', 'z'}; for (int i = 0; i < sizeof(chars)/sizeof(char); i++) { if (islower(chars[i])) { printf("%c is lowercase.\n", chars[i]); } else { printf("%c is not lowercase.\n", chars[i]); } } return 0; }
This snippet checks an array of characters, identifying which ones are lowercase. 'a' and 'z' will be recognized as lowercase, whereas 'B', '3', and '@' will not. The output reflects these findings clearly.
Conclusion
The islower()
function in C is a critical tool for verifying the case of alphabetic characters. It provides an efficient way to determine if a character is lowercase, simplifying many tasks in text processing and data validation. By integrating islower()
into your C programming toolset, you maintain robust and reliable text-based operations, thus ensuring your code adheres to necessary character case conditions. Use this function in varied scenarios where character case matters, boosting the effectiveness and efficiency of your programming efforts.
No comments yet.