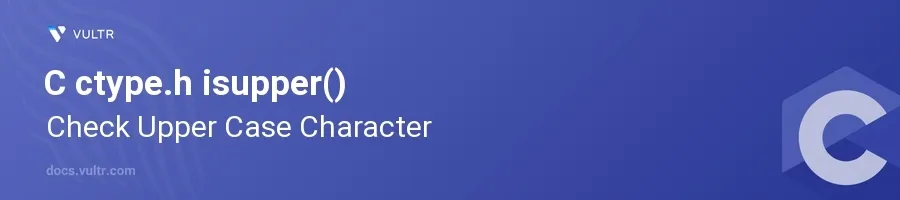
Introduction
The isupper()
function in C, provided by the ctype.h
library, serves as a handy tool to determine if a character is an uppercase letter. This function is extensively used in areas such as data validation, text processing, and user input handling, where character properties influence the program's behavior.
In this article, you will learn how to effectively utilize the isupper()
function in various scenarios. Explore how this function aids in validating text and implementing case-sensitive logic in C programming.
Understanding isupper()
Basic Usage of isupper()
Include the
ctype.h
header file which contains the declaration ofisupper()
.Pass a character to
isupper()
and check the returned value.c#include <ctype.h> #include <stdio.h> int main() { char ch = 'N'; if (isupper(ch)) { printf("%c is an uppercase letter.\n", ch); } else { printf("%c is not an uppercase letter.\n", ch); } return 0; }
In this code, you check if the character stored in
ch
is an uppercase letter. Ifisupper(ch)
returns a non-zero value, it indicates thatch
is uppercase. The program then prints a message confirming the character's case.
Handling Edge Cases
Test
isupper()
with different types of characters.Consider edge cases like numbers, symbols, and lowercase letters.
c#include <ctype.h> #include <stdio.h> void check_char(char ch) { if (isupper(ch)) { printf("%c is an uppercase letter.\n", ch); } else { printf("%c is not an uppercase letter.\n", ch); } } int main() { check_char('A'); // Expected: Uppercase check_char('z'); // Expected: Not uppercase check_char('5'); // Expected: Not uppercase check_char('@'); // Expected: Not uppercase return 0; }
This example extends the application of
isupper()
to diverse characters. By encapsulating the check into thecheck_char
function, it simplifies testing various characters to determine if they are uppercase letters.isupper()
effectively identifies only uppercase alphabetic characters, returning zero for all other types of characters.
Conclusion
The isupper()
function in C from ctype.h
is essential for distinguishing uppercase letters within a string or input. It simplifies many tasks related to text handling, like formatting or validating textual data. By mastering isupper()
, you enhance your ability to write clean, efficient, and robust C code that effectively handles and manipulates character data based on its case. Explore further by implementing this function in various text-processing applications to ensure your programs can dynamically respond to different types of character input.
No comments yet.