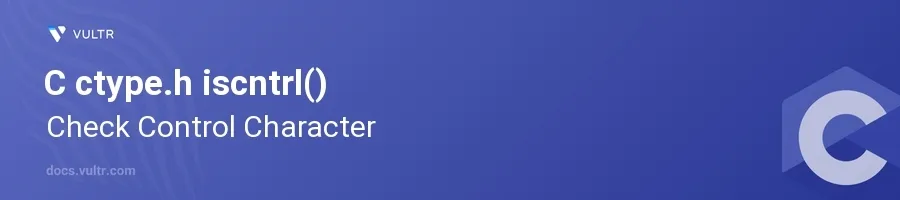
Introduction
The iscntrl()
function in C, part of the ctype.h
library, is a utility used for character classification. This function checks whether a given character is a control character such as a newline (\n
), carriage return (\r
), or a tab (\t
). Control characters are non-printable characters that do not represent symbols in a conventional character set.
In this article, you will learn how to use the iscntrl()
function effectively to identify control characters in C programming. The examples provided will illustrate the function's usage within various contexts, helping you to integrate it seamlessly into text processing tasks.
Understanding iscntrl()
Basic Usage of iscntrl()
Include the
ctype.h
header file in your program to use theiscntrl()
function.Pass the character to
iscntrl()
and evaluate the return value.c#include <stdio.h> #include <ctype.h> int main() { char c = '\n'; if (iscntrl(c)) { printf("The character is a control character.\n"); } else { printf("The character is not a control character.\n"); } return 0; }
This code checks if the character
c
(newline) is a control character. Since newline is a control character,iscntrl()
returns true, and the appropriate message is printed.
Applying iscntrl() in Text Processing
Define a string with diverse characters, including printable and control characters.
Iterate through each character of the string to test with
iscntrl()
.c#include <stdio.h> #include <ctype.h> int main() { char text[] = "Hello\nWorld\r"; for (int i = 0; text[i]; i++) { if (iscntrl(text[i])) { printf("Control character found: ASCII %d\n", text[i]); } } return 0; }
In this example, the string
text
contains both regular characters and control characters (\n
and\r
). The loop checks each character withiscntrl()
, and identifies the control characters by printing their ASCII values.
Conclusion
The iscntrl()
function from the ctype.h
library in C is an essential tool for character classification, particularly useful in scenarios where the identification of control characters is required. Whether parsing user inputs or processing textual data, the use of iscntrl()
helps ensure that control characters are appropriately handled or flagged. Implement this function in your text processing routines to maintain clear distinction between printable characters and control characters, enhancing both the reliability and readability of your C programs.
No comments yet.