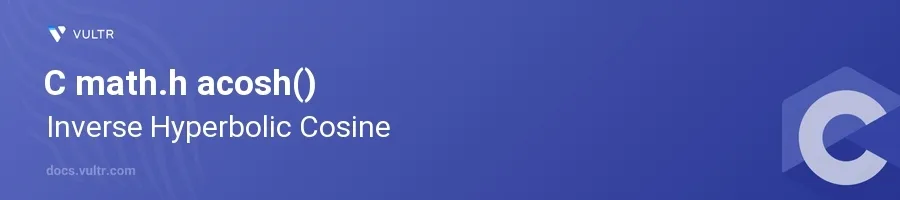
Introduction
The acosh()
function in C, provided by the math.h
library, computes the inverse hyperbolic cosine of a given number. This mathematical function is essential in various scientific, engineering, and financial calculations where modeling of natural logarithms is required for hyperbolic cosine values.
In this article, you will learn how to utilize the acosh()
function effectively. Understand the prerequisites of using this function, explore its application through practical code examples, and recognize its behavior with different inputs.
Understanding acosh() in C
Prerequisites for Using acosh()
Include the
math.h
header in your program to access theacosh()
function.Ensure the input value is a double type and greater than or equal to 1, as
acosh()
is defined for these values.C#include <math.h>
Including
math.h
enables access to various mathematical functions, includingacosh()
.
Basic Usage of acosh()
Declare and initialize a variable of type double with a value greater than or equal to 1.
Call the
acosh()
function with this variable as the argument.Store the result in a double variable and print it to verify the output.
C#include <stdio.h> #include <math.h> int main() { double value = 1.0; double result = acosh(value); printf("acosh(%.2f) = %.2f\n", value, result); return 0; }
This example calculates the inverse hyperbolic cosine of
1.0
. The expected output is0.00
becauseacosh(1)
is0
.
Advanced Scenarios with acosh()
Dealing with Larger Values
Apply
acosh()
to a larger value to see its effect on the outcome.Observe the rise in the return value as the input grows larger, reflecting the logarithmic nature of the inverse hyperbolic cosine function.
C#include <stdio.h> #include <math.h> int main() { double value = 10.0; double result = acosh(value); printf("acosh(%.2f) = %.2f\n", value, result); return 0; }
In this example, evaluating
acosh(10)
results in a larger output, demonstrating the logarithmic growth as inputs increase.
Error Handling
Understand that providing a value less than 1 results in a domain error because
acosh()
is not defined for such values in the real number system.C#include <stdio.h> #include <math.h> #include <errno.h> #include <fenv.h> int main() { double value = 0.5; double result = acosh(value); if (errno == EDOM) { printf("Error encountered: Input out of domain\n"); } else { printf("acosh(%.2f) = %.2f\n", value, result); } return 0; }
Here, attempting to compute
acosh(0.5)
triggers a domain error indicating the input is out of the function's domain.
Conclusion
The acosh()
function is a crucial component of the C standard library, enabling calculations of the inverse hyperbolic cosine. It is significant especially in fields requiring precise mathematical modeling. By handling inputs correctly and understanding the output, enhance your software's mathematical capabilities. Apply acosh()
in appropriate scenarios and manage potential errors to ensure robust and accurate computations in your programs.
No comments yet.