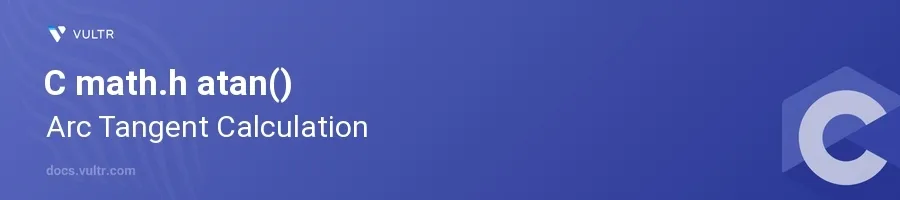
Introduction
The atan()
function in C, part of the math.h
header, computes the arc tangent of a given value. This trigonometric function is commonly used in geometrical calculations and scenarios where you need to determine angles from the ratio of two sides of a right triangle.
In this article, you will learn how to effectively apply the atan()
function in various programming contexts. Explore its essential role in calculating angles and its usage patterns in both simple and complex mathematical computations.
Understanding atan()
Basic Usage of atan()
Include the
math.h
header file in your program to useatan()
.Declare a variable and assign it a value whose arc tangent you want to calculate.
Call
atan()
passing this value as an argument and assign the result to another variable to store the angle in radians.c#include <math.h> #include <stdio.h> int main() { double x = 1.0; double angle_rad = atan(x); printf("The arc tangent of %f is %f radians\n", x, angle_rad); return 0; }
This code calculates the arc tangent of
1.0
. As the tangent of 45 degrees or π/4 radians is1.0
,atan(1.0)
returns π/4, which is approximately 0.785398.
Applying atan() in Coordinate Geometry
Use
atan()
to find the angle of a line relative to the x-axis.Define a coordinate point
(x, y)
, not on the origin.Compute the angle using
atan(y/x)
.c#include <math.h> #include <stdio.h> int main() { double x = 1.0, y = 1.0; double angle_rad = atan(y / x); printf("The angle of the line is %f radians\n", angle_rad); return 0; }
Here, for a line from the origin
(0,0)
to point(1,1)
, the calculated angle of the line is π/4 radians, reflecting its 45-degree angle with respect to the x-axis.
Beyond Basics: Complex Use Cases
Combining atan() with Other Mathematical Functions
To handle complex scenarios like determining direction or angle differences, combine
atan()
with functions likesin()
andcos()
.For a moving object's trajectory, calculate the difference in angle based on velocity vectors.
c#include <math.h> #include <stdio.h> int main() { double velocity_x = 5.0, velocity_y = 5.0; double angle_rad = atan(velocity_y / velocity_x); double direction_cosine = cos(angle_rad); printf("Direction Cosine: %f\n", direction_cosine); return 0; }
This example shows how to use
atan()
in combination withcos()
to find the direction cosine of the velocity vector.
Conclusion
Understanding and utilizing the atan()
function from math.h
in C provides a powerful tool for computing arc tangents and angles in various geometric and physical computation scenarios. Whether dealing with basic angles or complex kinematic calculations, atan()
serves as an essential component in angle-related computations. Familiarize with its functionality and integration with other math functions to enhance the computational capabilities of your C programs.
No comments yet.