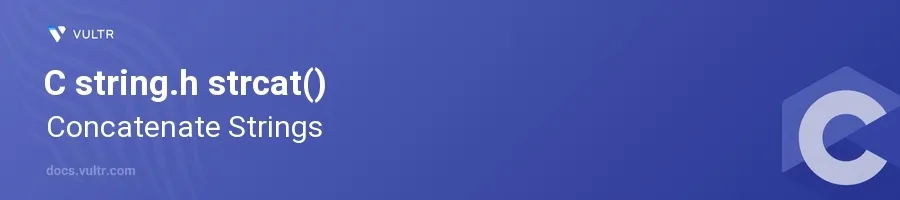
Introduction
The strcat()
function is a standard library function in C, found within the <string.h>
header, used to concatenate two strings. This function appends the content of one string to another, effectively joining them into a single string. The operation modifies the destination string and is a straightforward, yet crucial tool in C programming for handling text data.
In this article, you will learn how to effectively use the strcat()
function to concatenate strings. Discover how it functions with various examples, and understand the scenarios where it is appropriate to implement this method.
Basic Usage of strcat()
Append String to Another
Include the
<string.h>
library to accessstrcat()
.Declare and initialize two strings: a destination and a source string.
Apply
strcat()
to append the source string to the destination string.c#include <string.h> #include <stdio.h> int main() { char dest[20] = "Hello"; char src[] = " World"; strcat(dest, src); printf("Concatenated String: %s\n", dest); return 0; }
This code first initializes the
dest
array with a size sufficient to hold the concatenated result. Thestrcat()
function then appends the content ofsrc
todest
, resulting indest
containing "Hello World".
Understanding Buffer Sizes
Always ensure that the destination string has enough space to hold the concatenated result.
Calculate the required size before performing concatenation to avoid buffer overflows.
c#include <string.h> #include <stdio.h> int main() { char dest[11] = "Hello"; char src[] = " World"; if (sizeof(dest) >= strlen(dest) + strlen(src) + 1) { strcat(dest, src); printf("Safe Concatenation: %s\n", dest); } else { printf("Not enough space in 'dest' to concatenate 'src'\n"); } return 0; }
The check ensures that there is enough space in
dest
for the concatenated strings and the null terminator. This is critical to prevent buffer overflow, which can lead to undefined behavior and security vulnerabilities.
Advanced Uses of strcat()
Concatenating Multiple Strings
Declare and initialize several source strings.
Use multiple
strcat()
calls to append each string to the destination.c#include <string.h> #include <stdio.h> int main() { char dest[50] = "Hello"; char src1[] = ", how"; char src2[] = " are you?"; char src3[] = " Doing well!"; strcat(dest, src1); strcat(dest, src2); strcat(dest, src3); printf("Full Message: %s\n", dest); return 0; }
Each call to
strcat()
appends the subsequent source string todest
, gradually building up the complete message. Remember to maintain a buffer large enough to accommodate all appendages.
Conclusion
The strcat()
function from the <string.h>
library is indispensable for string concatenation in C programming. It is simple to use but requires careful management of buffer size to ensure memory safety. Use this function to combine strings effectively, whether you are generating messages, processing textual data, or configuring complex string constructs. Through the practices outlined in this article, enhance the reliability and safety of your text manipulation tasks in C.
No comments yet.