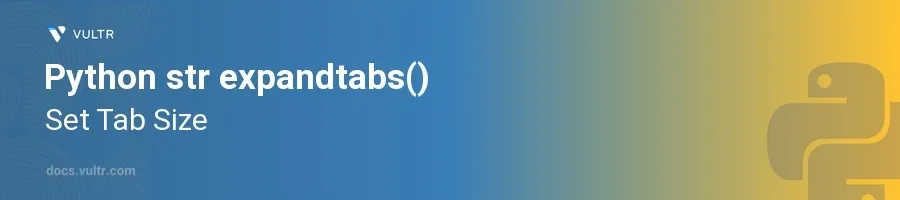
Introduction
The expandtabs()
method in Python is a string method used for setting the tab size in a given string. This method returns a copy of the string where all tab characters (\t
) are replaced with the right amount of space, based on the tab size specified. This feature is particularly useful for formatting output in console applications where consistent column spacing is required.
In this article, you will learn how to effectively use the expandtabs()
method to manage and modify string outputs. Discover the capabilities of this method by exploring a variety of examples that illustrate how to control and adjust tab spaces for better text alignment and readability.
Basic Usage of expandtabs()
Replacing Tabs with Spaces
Start with a simple string containing tab characters.
Use the
expandtabs()
method to replace the tabs.pythontext = "Python\tis\ta\tgreat\tlanguage." print("Original Text:", text) formatted_text = text.expandtabs(8) print("Formatted Text:", formatted_text)
In this example, each tab character (
\t
) in the string is replaced by eight spaces, which is the default tab size if no parameter is provided. The output shows how the text alignment changes when tabs are expanded.
Custom Tab Size
Use a string that includes tabs.
Apply
expandtabs()
with a custom tab size of your choice.pythontext = "Item\tPrice\tQuantity\nPens\t$1.20\t10" formatted_text = text.expandtabs(12) print(formatted_text)
Here, the tab size is set to 12. This makes each tab character expand to 12 spaces, allowing for wider spacing between columns. This can be particularly useful for making table-like structures more readable.
Advanced Usage of expandtabs()
Ensuring Column Alignment in Data Output
Prepare a multiline string simulating a simple data table format.
Choose an appropriate tab size to align all columns evenly.
Apply
expandtabs()
to enhance readability.pythondata = "Name\tAge\tCity\nJohn Doe\t28\tNew York\nJane Smith\t32\tLos Angeles" aligned_data = data.expandtabs(16) print(aligned_data)
This code snippet demonstrates how
expandtabs()
can be used to align columns in a string that represents tabular data. By expanding tabs to a specified number of spaces, each column starts at a consistent horizontal position, enhancing the data's readability.
String List Manipulation for Dynamic Tab Sizes
Create a list of strings where each item contains tab characters.
Determine the optimal tab size based on the maximum string length in the list.
Iterate through the list, applying
expandtabs()
to each string.pythonnames = ["Alice\tAnderson", "Bob\tBrown", "Charlotte\tCunningham"] max_length = max(len(name.split('\t')[0]) for name in names) tab_size = max_length + 4 # Adding 4 spaces for cushion adjusted_names = [name.expandtabs(tab_size) for name in names] for name in adjusted_names: print(name)
By calculating the maximum length of the first column dynamically and setting the tab size accordingly, the
expandtabs()
method ensures that the second column is perfectly aligned across all items. This approach is highly flexible and adapts to varying lengths of string data.
Conclusion
The expandtabs()
function in Python provides a practical and efficient way of handling and formatting strings containing tab characters. Whether aligning data columns in output, creating organized table-like structures, or simply adjusting tab spaces for better readability, this method offers a reliable solution. Apply the techniques discussed here to enhance the appearance and readability of your string outputs, ensuring that your data presentations are both clear and professional.
No comments yet.