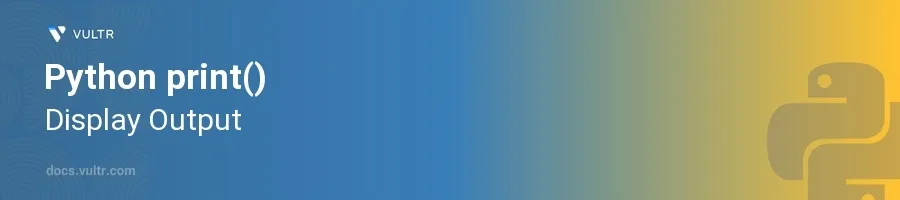
Introduction
The print()
function in Python is fundamental for outputting data to the console, making it an indispensable tool for debugging and displaying information. This simple yet powerful function allows for formatting and directing output in versatile ways, which is essential in both simple scripts and complex applications.
In this article, you will learn how to effectively utilize the print()
function to display various types of data in Python. You will explore different techniques for formatting output, managing multiple data items, and directing output streams.
Basic Usage of print()
Displaying a Simple Message
Use
print()
to output a simple text message.pythonprint("Hello, world!")
This code prints the string
"Hello, world!"
to the console.
Outputting Multiple Items
Pass multiple arguments to
print()
to display them in a single line separated by spaces.pythonprint("The answer is", 42)
Here,
print()
combines the string"The answer is"
and the number42
, separating them with a space before outputting the combined message to the console.
Formatting Output
Using String Concatenation
Concatenate strings and variables before passing them to
print()
.pythonname = "Alice" print("Hello, " + name + "!")
Concatenation combines the greeting
"Hello, "
with the variablename
holding"Alice"
, and an exclamation point, resulting in the output"Hello, Alice!"
.
Utilizing String Formatting
Use
str.format()
for a cleaner way to format complex strings.pythonage = 30 print("I am {} years old".format(age))
This snippet uses
{}
as a placeholder for the variableage
within the string. Whenprint()
executes, it replaces{}
with the value ofage
, resulting in"I am 30 years old"
.
Employing f-Strings for Formatting (Python 3.6+)
Leverage f-strings for a more intuitive and readable way to insert variables into strings.
pythonscore = 95 print(f"Your score is {score}%")
F-strings allow embedding expressions inside string literals directly, making the code concise and easy to understand. The
score
variable is directly placed within the string.
Advanced Techniques
Changing the Separator between Items
Control how
print()
separates multiple items with thesep
parameter.pythonprint("Earth", "Mars", "Venus", sep=", ")
Instead of the default space, this code uses a comma followed by a space to separate the items, outputting
"Earth, Mars, Venus"
.
Redirecting the Output
Redirect the output of
print()
to a file instead of the console.pythonwith open("output.txt", "w") as file: print("Hello, file!", file=file)
This code directs the output of
print()
into a file named"output.txt"
, writing"Hello, file!"
into it instead of printing on the console.
Ending the Output Differently
Customize the end character of the
print()
function using theend
parameter.pythonprint("Hello", end="!") print("World")
The first
print()
ends with an exclamation mark, resulting in the output"Hello!World"
on the same line, demonstrating how theend
parameter can modify the default newline character.
Conclusion
The print()
function in Python offers extensive functionality for displaying output in different formats and destinations. By mastering the varied capabilities of print()
, from simple data output to advanced formatting techniques, you can make debugging easier and enhance the clarity of information presented to users or during development. Implement these techniques to fine-tune how data is displayed and make your Python scripts more effective and user-friendly.
No comments yet.