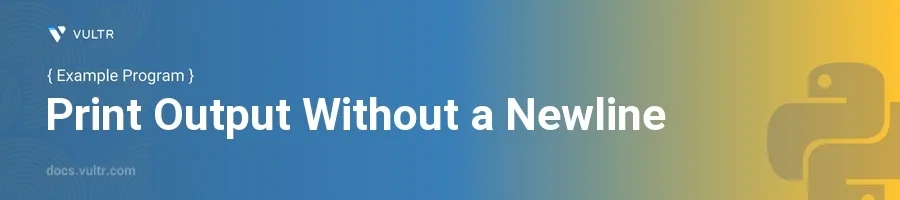
Introduction
In Python, printing output typically ends with a newline character, which means each print statement adds its output on a new line. This behavior is useful in many contexts but can sometimes be restrictive when you want to continue a message or data output on the same line.
In this article, you will learn how to control the print behavior in Python to avoid the automatic newline at the end of the output. Discover various ways to tailor the print function for formatting output without starting a new line each time you issue a print command. This knowledge is particularly useful in creating customized outputs, such as progress bars, inline lists, or continuous data streams.
Advanced Print Function Usage
Controlling End Character in Print Function
Use the
print()
function’send
parameter to control what character is printed at the end of the statement, instead of the default newline character (\n
).Modify the
end
parameter to an empty string (''
) if no ending is desired.pythonprint("Hello,", end=" ") print("world!")
In this code, "Hello," is printed followed by a space (instead of a newline) and then "world!" is printed. The output on the screen will be
Hello, world!
.
Using a Custom End Character
Customize the
end
parameter to suit various formatting needs other than removing the newline completely.Set a different ending character like a period, dash, or any symbol.
pythonprint("Loading", end="...") print("Complete")
Here, the output will be displayed as
Loading...Complete
on the same line. This technique is particularly effective for status updates or loading messages.
Creating Continuous Outputs
Printing Values from a Loop on the Same Line
Use the
end
parameter within loops to print all iterations on a single line.Combine
end
with spacing or other characters to format output neatly.pythonfor i in range(5): print(i, end=" ")
The output of this code will be
0 1 2 3 4
, which demonstrates how loop iterations can be printed inline without line breaks.
Using Print to Create a Progress Bar
Simulate a progress bar by printing a single character repeatedly without a newline.
Employ a slight delay to enhance the effect of the progress bar.
pythonimport time for i in range(10): print('*', end='', flush=True) time.sleep(0.5) # Delays for half a second
This code snippet prints a star every half second on the same line, creating a progressing bar effect. The
flush=True
ensures that each output is immediately visible.
Conclusion
Mastering the use of the print()
function without a newline in Python opens up numerous opportunities for output customization and formatting. From simply changing the end character to constructing complex same-line outputs within loops or simulating dynamic effects like progress bars, control over output formatting is a valuable skill. Implement these techniques in your scripts to enhance their functionality and improve the user experience by providing clear and custom formatted outputs.
No comments yet.