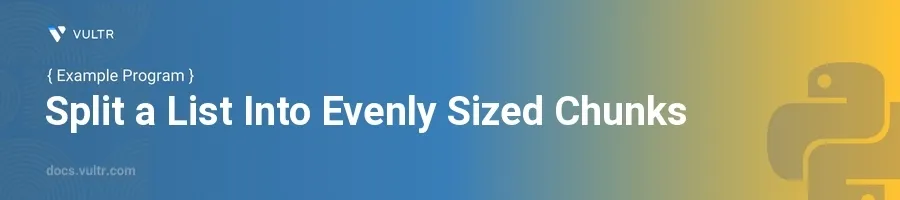
Introduction
Splitting large lists into smaller sub-lists or chunks in Python can make the lists easily manageable. This is useful in data processing where operations need to be batched to optimize performance, or when sending data over a network and need to adhere to size constraints. Splitting a list into chunks in Python can also help in parallel processing scenarios where tasks can be distributed across multiple threads or processes efficiently.
In this article, you will learn how to split a list into evenly sized chunks using Python. You will explore several methods to achieve this, using simple Python functions, list comprehensions, and generators. By the end of this article, you will be able to implement splitting functionality in Python efficiently in your projects, aiding in various scenarios such as data batching, parallel computation, and more.
Basic Loop Method to Split Lists into Chunks in Python
Splitting Lists into Chunks Using a Simple Iterative Approach
This method demonstrates how to split a list into equal parts in Python using a straightforward loop-based technique.
Define your list and the size of each chunk.
Initialize an empty list to hold the chunks.
Use a loop to create and append each chunk to the resulting list.
pythondef split_list_simple(my_list, chunk_size): chunks = [] for i in range(0, len(my_list), chunk_size): chunks.append(my_list[i:i + chunk_size]) return chunks # Example usage example_list = [1, 2, 3, 4, 5, 6, 7, 8, 9] result = split_list_simple(example_list, 4) print(result)
This function iterates over the original list and creates a new chunk on each iteration by slicing the list from the current index
i
toi + chunk_size
. The slices are then appended to thechunks
list. In the example provided, the Python list is split into chunks where each chunk (except possibly the last) has 4 elements.
List Comprehension in Python for Efficient Chunking
Using List Comprehension for a More Concise Solution
Reimplement the splitting functionality using list comprehension, which can make the code more concise and potentially more readable.
Define the list and the desired chunk size as before.
pythondef split_list_comprehension(my_list, chunk_size): return [my_list[i:i + chunk_size] for i in range(0, len(my_list), chunk_size)] # Example usage example_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] result = split_list_comprehension(example_list, 3) print(result)
In this method, a list comprehension is used to achieve the same result as the simple iterative approach but in a more compact form. The comprehension iterates over the original list in steps of
chunk_size
and slices the list accordingly. The function returns these slices directly as a list of chunks.
Using Generators to Split Large Lists into Chunks in Python
Implementing a Generator Function to Handle Large Data Efficiently
Consider using a generator if the list is extremely large or if you want to handle chunks one at a time without loading all chunks into memory.
Implement a generator that yields each chunk sequentially.
pythondef split_list_generator(my_list, chunk_size): for i in range(0, len(my_list), chunk_size): yield my_list[i:i + chunk_size] # Example usage example_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12] for chunk in split_list_generator(example_list, 4): print(chunk)
This Python generator function uses the same logic as earlier methods but leverages the
yield
statement to return one chunk at a time. It is ideal when you want to partition a list into chunks without keeping all chunks in memory. Use this when processing data streams or working with very large lists.
Conclusion
Splitting a list into chunks in Python can be accomplished through multiple techniques such as basic loops, list comprehensions, and generators. Choosing the right method depends on your specific use case, whether it's about memory efficiency, code readability, or handling large datasets. Whether you're aiming to chunk an array in Python, split a list into equal parts, or divide a list into batches, these Pythonic methods will serve you well. Mastering these approaches will enable you to efficiently chunk Python lists, making your code cleaner and more scalable across a range of data processing scenarios.
No comments yet.