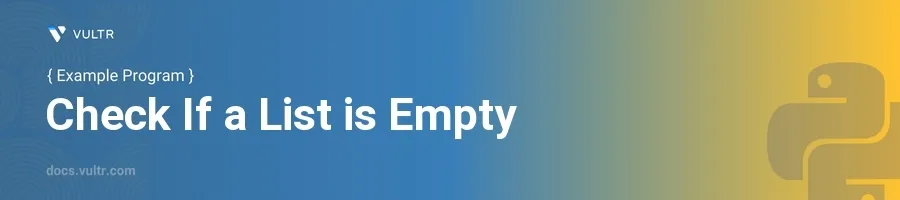
Introduction
Checking if a list is empty is a common task in Python programming, especially when dealing with data that varies in content. An empty list can signify that data is missing, not generated, or has been entirely consumed by previous operations. Determining whether a list is empty helps in controlling the flow of logic in programs, allowing for the implementation of conditional statements that alter behavior based on data availability.
In this article, you will learn how to check if a list is empty through various Python examples. These examples will cover straightforward methods and provide insights on optimizing code readability and performance.
Checking for Emptiness Directly
Using the Boolean Context
Recognize that empty lists evaluate to
False
in a boolean context.Directly use the list in an
if
orif not
statement to check if it's empty.pythonmy_list = [] if not my_list: print("List is empty.") else: print("List is not empty.")
Here,
if not my_list
effectively checks ifmy_list
is empty and prints "List is empty." Otherwise, it prints "List is not empty."
Comparing Against an Empty List
Using the Equality Operator
Compare the list directly to an empty list
[]
using the==
operator.This method makes the check explicit and clear in the code.
pythonmy_list = [] if my_list == []: print("List is empty.") else: print("List is not empty.")
The condition
my_list == []
comparesmy_list
with an empty list and executes the print statement accordingly.
Utilizing the len() Function
Checking List Length
Use the
len()
function to determine the number of items in the list.An empty list has a length of
0
, which can be checked directly.pythonmy_list = [] if len(my_list) == 0: print("List is empty.") else: print("List is not empty.")
The
len(my_list) == 0
condition will beTrue
ifmy_list
is empty, triggering the print statement.
Conclusion
Checking if a list is empty in Python can be performed in several ways, each serving different readability and contextual needs. Using direct boolean evaluation is typically the simplest and most performant. However, explicitly comparing against an empty list or using the len()
function might clarify intent in contexts where code readability is paramount. Choose the method that best fits the scenario to keep your Python code clean and efficient. Each technique discussed here ensures your program handles data conditions appropriately, improving its robustness and reliability.
No comments yet.