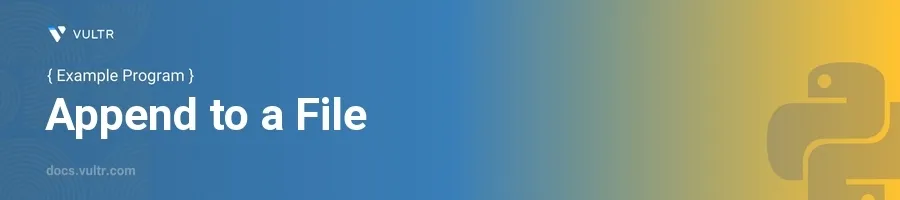
Introduction
Appending to a file in Python is a common file operation used to add content to the end of an existing file without overwriting the original content. This technique is helpful for logging, data accumulation over time, or simply extending file data in various applications.
In this article, you will learn how to append text to a file in Python using different approaches and functionalities provided by Python's file handling methods. Explore practical examples that demonstrate how to implement appending operations effectively in your programs.
Appending Text to a File Using open()
Basic Text Appending
Open the file in append mode by setting the mode to
'a'
. If the file doesn't exist, Python creates it.Use the
write()
method to add text to the file.pythonwith open('example.txt', 'a') as file: file.write("Appending text to the file.\n")
This code opens
example.txt
in append mode and writes a line of text to it. Each execution of this code appends the text again at the end of the file.
Appending with New Lines
Ensure each appended text starts on a new line.
Use the newline character
\n
to separate lines effectively.pythonwith open('example.txt', 'a') as file: file.write("First line of text.\n") file.write("Second line of text.\n")
The newline
\n
at the end of each string ensures that each call towrite()
starts at a new line in the file.
Using print()
Function for Appending
Appending Multiple Items Conveniently
Make use of the
print()
function, which can handle multiple arguments and automatic newline insertion.Open the file with the
'a'
mode.pythonwith open('example.txt', 'a') as file: print("Appending new data.", "Additional text.", sep='\n', file=file)
The
print()
function is used here to append text. Thesep='\n'
argument ensures each output is on a new line. This method is beneficial for appending multiple items with custom separations.
Appending with Context Managers
Ensuring Safe File Operations
Use a context manager (
with
statement) to handle file operations securely.Automatically manage file closing and error handling.
pythonwith open('example.txt', 'a') as file: file.writelines(["First new line.\n", "Second new line.\n"])
Using
writelines()
with a list of strings appends each string to the file. Unlikewrite()
,writelines()
does not automatically add newline characters, so they need to be included in the strings.
Conclusion
Appending to a file in Python is straightforward with the use of the open()
function in append mode. Whether using the simple write()
method, leveraging the convenience of the print()
function, or ensuring robust file handling with context managers, Python offers versatile ways to add content to files effectively. These techniques can be crucial for applications that require data logging, iterative data append, or simple file content extension. Utilize these methods in your scripts to enhance the functionality and maintainability of your file operations.
No comments yet.