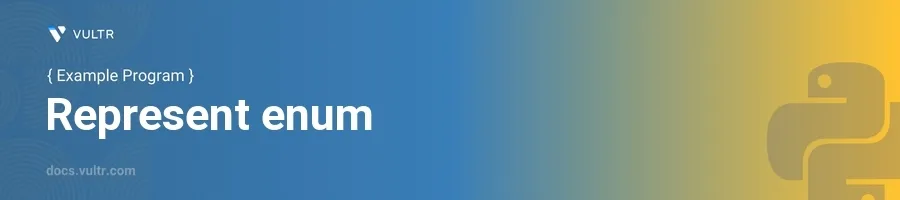
Introduction
Enums, short for enumerations, are sets of symbolic names bound to unique, constant values. In Python, enums make code more readable and maintainable by providing meaningful labels instead of using magic numbers. They are commonly used in scenarios where a variable can have one out of a set of predefined constants.
In this article, you will learn how to effectively leverage the enum
module to create enums in Python. Discover the implementation of enums through examples, understand how they can clean up your code, and explore various functionalities that the module provides such as value comparisons, iteration, and automatic value assignment.
Basic Usage of Enums in Python
Creating an Enum
Import the Enum class.
Define an Enum by sub-classing Enum.
pythonfrom enum import Enum class Color(Enum): RED = 1 GREEN = 2 BLUE = 3
In this code snippet, an enum
Color
is defined with three members:RED
,GREEN
, andBLUE
. Each member is associated with a numeric value.
Accessing Enum Members
Access enum members using their names.
Print the member and its type to understand its structure.
pythonprint(Color.RED) print(type(Color.RED))
This snippet demonstrates how to access and print an enum member along with its type. The output will show both the name and value of the enum member.
Enumeration Members' Types and Values
Access the name and value of enumeration members.
Display these properties.
pythonprint("Name:", Color.RED.name) print("Value:", Color.RED.value)
Here,
Color.RED.name
andColor.RED.value
will outputRED
and1
, respectively. These attributes are particularly useful for debugging and logging purposes.
More Advanced Enum Features
Auto-numbering with auto()
Use the
auto()
function to automatically assign values.Avoid manually assigning values to each member.
pythonfrom enum import Enum, auto class Color(Enum): RED = auto() GREEN = auto() BLUE = auto()
This example avoids manually specifying the values for each enum member. The
auto()
function automatically assigns incremental integers starting from 1.
Iteration Over Enums
Iterate through all members of an enum.
Print each member's name and value.
pythonfor color in Color: print(f"{color.name} has value {color.value}")
By iterating over
Color
, you can access each member’s name and value in a loop, which is useful for scenarios where you need to display or process a list of all members in an enum.
Enums with Functions
Define methods inside an enum.
Use Enums in a more functional way.
pythonclass Color(Enum): RED = 1 GREEN = 2 BLUE = 3 def description(self): if self == Color.RED: return "This color is red." elif self == Color.GREEN: return "This color is green." elif self == Color.BLUE: return "This color is blue."
description
is a method that returns a string explaining the color. This example adds more functionality to the enum, making it not just a namespace but also an object capable of carrying behavior.
Conclusion
Python's enum module provides a structured way to handle sets of constants. By using enums, maintain clean and readable code, and reduce the number of magic numbers in your codebase. Enums enhance code readability, ensure code robustness, and facilitate easier debugging and maintenance. With the techniques discussed, employ enums to define a coherent set of constants that can make your programming more intuitive and error-free. Whether it’s simple value assignments or incorporating functions, enums prove to be incredibly versatile and powerful in organizing and managing predefined constants in Python applications.
No comments yet.